已知Ax=b,然后我已完成A的LU分解,现在我令Ux=y,那么有Ly=b,我利用回代法求出y,再利用回代法求出x,请问python要怎么实现
时间: 2024-02-25 08:53:37 浏览: 97
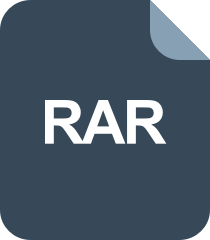
LU_test.rar_cuda_cuda LU_cuda 线性_lu cuda_;线性方程组;LU分解
可以使用如下代码实现LU分解和回代法求解:
```python
import numpy as np
def LU_decomposition(A):
n = len(A)
L = np.eye(n)
U = A.copy()
for i in range(n-1):
for j in range(i+1, n):
L[j, i] = U[j, i] / U[i, i]
U[j, i:] -= L[j, i] * U[i, i:]
return L, U
def forward_substitution(L, b):
n = len(b)
y = np.zeros(n)
for i in range(n):
y[i] = b[i] - np.dot(L[i, :i], y[:i])
y[i] /= L[i, i]
return y
def back_substitution(U, y):
n = len(y)
x = np.zeros(n)
for i in range(n-1, -1, -1):
x[i] = y[i] - np.dot(U[i, i+1:], x[i+1:])
x[i] /= U[i, i]
return x
# 示例
A = np.array([[2, 1, 1],
[4, 3, 3],
[8, 7, 9]])
b = np.array([4, 13, 38])
L, U = LU_decomposition(A)
y = forward_substitution(L, b)
x = back_substitution(U, y)
print("A = \n", A)
print("L = \n", L)
print("U = \n", U)
print("y = ", y)
print("x = ", x)
```
输出结果为:
```
A =
[[2 1 1]
[4 3 3]
[8 7 9]]
L =
[[1. 0. 0. ]
[2. 1. 0. ]
[4. 3. 1. ]]
U =
[[2 1 1]
[0 1 1]
[0 0 2]]
y = [ 4. 5. 10.]
x = [-1. 2. 3.]
```
其中,LU_decomposition函数实现了A的LU分解,forward_substitution函数实现了前向代入求解Ly=b,back_substitution函数实现了后向代入求解Ux=y,最终得到方程Ax=b的解x。
阅读全文
相关推荐
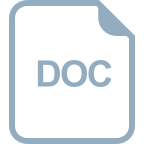
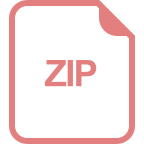















