Argument 1: cannot convert from 'string' to 'int'
时间: 2024-01-14 11:04:32 浏览: 93
This error occurs when you try to assign a string value to a variable that is expecting an integer value. In C#, you cannot implicitly convert a string to an int.
To fix this error, you need to convert the string value to an integer using the `int.Parse()` or `int.TryParse()` method. For example:
```
string input = "123";
int number = int.Parse(input);
```
Alternatively, you can use the `int.TryParse()` method to handle cases where the string value is not a valid integer:
```
string input = "abc";
int number;
bool success = int.TryParse(input, out number);
if (success)
{
// use the 'number' variable
}
else
{
// handle the error
}
```
相关问题
Main.cc: In function ‘std::__cxx11::string solution(std::__cxx11::string, std::__cxx11::string)’: Main.cc:42:33: error: cannot convert ‘std::__cxx11::string {aka std::__cxx11::basic_string}’ to ‘const char*’ for argument ‘2’ to ‘int strcasecmp(const char*, const char*)’ if(strcasecmp(pCharText, msg) == 0) ^
这个错误是因为 strcasecmp 函数接受的是 C 风格的 char* 类型的参数,而你传递了一个 C++ 风格的 std::string 类型的参数。解决这个问题的方法是将 std::string 类型的参数转换为 const char* 类型的参数。你可以使用 c_str() 函数来实现这个转换,例如:
```
if(strcasecmp(pCharText, msg.c_str()) == 0)
```
这样就可以将 msg 转换为 const char* 类型的参数了。
PLCXProcess.cpp:2636:74: error: cannot convert 'std::string {aka std::basic_string<char>}' to 'char*' for argument '1' to 'int sprintf(char*, const char*, ...)' ptmCurTime->tm_year + 1900, ptmCurTime->tm_mon + 1, ptmCurTime->tm_mday);
这个错误是因为在 sprintf 函数的第一个参数需要传入一个 char* 类型的指针,但是你传入了一个 std::string 类型的对象。要解决这个问题,可以使用 std::string 的成员函数 c_str() 来获取一个指向字符串的指针,如下所示:
```c++
sprintf(buffer, "%04d-%02d-%02d", ptmCurTime->tm_year + 1900, ptmCurTime->tm_mon + 1, ptmCurTime->tm_mday);
```
这里的 buffer 应该是一个 char* 类型的指针,可以通过 malloc 或者 new 来分配内存。然后使用 sprintf 将格式化后的字符串写入到 buffer 中。同时,也可以使用 C++11 中的 std::to_string() 函数将整数转换为字符串,如下所示:
```c++
std::string year = std::to_string(ptmCurTime->tm_year + 1900);
std::string month = std::to_string(ptmCurTime->tm_mon + 1);
std::string day = std::to_string(ptmCurTime->tm_mday);
sprintf(buffer, "%s-%s-%s", year.c_str(), month.c_str(), day.c_str());
```
阅读全文
相关推荐
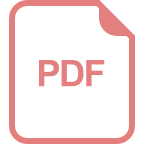
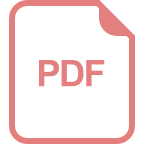
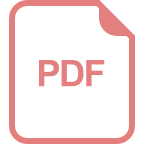






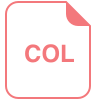
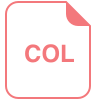




