cannot convert 'ElemType {aka STU}' to 'const char*' for argument '2' to 'int strcmp(const char*, const char*)'
时间: 2023-12-25 19:03:54 浏览: 138
这个错误是因为你在使用strcmp函数时,第二个参数应该是const char*类型,但你传入的是ElemType类型。你需要将ElemType类型转换成const char*类型。
例如,如果你想比较ElemType类型的name成员变量与一个字符串是否相等,你可以这样写:
```c++
if (strcmp(elem.name.c_str(), "some string") == 0) {
// do something
}
```
这里使用了string类的c_str()函数将string类型转换成const char*类型。你需要根据实际情况来修改代码。
相关问题
error: cannot convert 'ElemType*' to 'const char*'|
这个错误是因为在程序中将 `ElemType*` 类型的指针转换为了 `const char*` 类型,而这两种类型并不是直接可以转换的。
可能的原因是在定义 `ElemType` 类型时没有指定其具体类型,导致在程序中无法正确地使用字符类型的变量和字符串常量。
为了解决这个问题,可以检查以下几个方面:
1. 在定义 `ElemType` 类型时,需要明确它的具体类型,例如可以这样定义:
```
typedef char ElemType;
```
或者
```
typedef string ElemType;
```
这样就可以正确地使用字符类型或字符串类型的变量和字符串常量。
2. 在程序中使用字符类型的变量和字符串常量时,需要注意它们的类型,不能将 `ElemType*` 类型的指针转换为 `const char*` 类型。
如果 `ElemType` 的类型是 `char`,那么可以直接使用字符变量和字符串常量,例如:
```
ElemType c = 'a';
const ElemType* s = "hello";
```
如果 `ElemType` 的类型是 `string`,那么需要使用 `c_str()` 函数将 `string` 类型的变量或字符串常量转换为 `const char*` 类型,例如:
```
ElemType str = "hello";
const ElemType* s = str.c_str();
```
在程序中使用 `s` 时,需要注意它的类型为 `const ElemType*`,不能将其转换为 `const char*` 类型。
希望这些解释可以帮助你解决问题。
error: cannot convert 'char*' to 'ElemType*'|
这个错误通常是因为在使用函数的时候,传入的参数类型不正确,导致编译器无法正常进行类型转换。
例如,当函数期望的参数类型为 ElemType*,而你传入的实参类型为 char*,就会发生这个错误。
解决方法是需要将参数类型进行转换,确保传入的参数类型与函数期望的参数类型一致。
示例代码:
```c++
#include <iostream>
#include <cstring>
using namespace std;
typedef char ElemType; // 定义 ElemType 类型为 char
void printArray(ElemType arr[], int len) { // 使用 ElemType 类型作为参数类型
for (int i = 0; i < len; i++) {
cout << arr[i] << " ";
}
cout << endl;
}
int main() {
char str[] = "Hello World!";
int len = strlen(str);
printArray(str, len); // 传入参数时,需要将 char[] 类型的 str 转换为 ElemType[] 类型
return 0;
}
```
输出结果为:
```
H e l l o W o r l d !
```
阅读全文
相关推荐
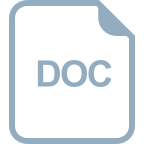
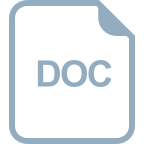
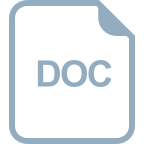













