python海龟作图经典100例
时间: 2023-07-07 20:34:00 浏览: 169
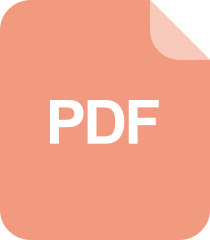
python海龟绘图实例教程

好的,以下是Python海龟作图经典100例:
1. 绘制一个正方形
```python
import turtle
turtle.forward(100)
turtle.right(90)
turtle.forward(100)
turtle.right(90)
turtle.forward(100)
turtle.right(90)
turtle.forward(100)
```
2. 绘制一个圆形
```python
import turtle
turtle.circle(50)
```
3. 绘制一个五角星
```python
import turtle
turtle.forward(100)
turtle.right(144)
turtle.forward(100)
turtle.right(144)
turtle.forward(100)
turtle.right(144)
turtle.forward(100)
turtle.right(144)
turtle.forward(100)
```
4. 绘制一个正方形螺旋
```python
import turtle
for i in range(100):
turtle.forward(i)
turtle.right(90)
```
5. 绘制一个彩色螺旋
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
for i in range(100):
turtle.color(colors[i % 6])
turtle.forward(i)
turtle.right(90)
```
6. 绘制一个三角形
```python
import turtle
turtle.forward(100)
turtle.left(120)
turtle.forward(100)
turtle.left(120)
turtle.forward(100)
```
7. 绘制一个五边形
```python
import turtle
turtle.forward(100)
turtle.right(72)
turtle.forward(100)
turtle.right(72)
turtle.forward(100)
turtle.right(72)
turtle.forward(100)
turtle.right(72)
turtle.forward(100)
```
8. 绘制一个六边形
```python
import turtle
turtle.forward(100)
turtle.right(60)
turtle.forward(100)
turtle.right(60)
turtle.forward(100)
turtle.right(60)
turtle.forward(100)
turtle.right(60)
turtle.forward(100)
turtle.right(60)
turtle.forward(100)
```
9. 绘制一个正方形螺旋(使用for循环)
```python
import turtle
for i in range(100):
turtle.forward(i * 2)
turtle.right(90)
```
10. 绘制一个彩色螺旋(使用for循环)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
for i in range(100):
turtle.color(colors[i % 6])
turtle.forward(i * 2)
turtle.right(90)
```
11. 绘制一个五角星(使用for循环)
```python
import turtle
for i in range(5):
turtle.forward(100)
turtle.right(144)
```
12. 绘制一个正方形(使用for循环)
```python
import turtle
for i in range(4):
turtle.forward(100)
turtle.right(90)
```
13. 绘制一个圆形(使用for循环)
```python
import turtle
for i in range(360):
turtle.forward(1)
turtle.right(1)
```
14. 绘制一个三角形(使用for循环)
```python
import turtle
for i in range(3):
turtle.forward(100)
turtle.left(120)
```
15. 绘制一个六边形(使用for循环)
```python
import turtle
for i in range(6):
turtle.forward(100)
turtle.right(60)
```
16. 绘制一个彩色正方形螺旋(使用for循环)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
for i in range(100):
turtle.color(colors[i % 6])
turtle.forward(i * 2)
turtle.right(90)
```
17. 绘制一个彩色五角星(使用for循环)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
for i in range(5):
turtle.color(colors[i % 6])
turtle.forward(100)
turtle.right(144)
```
18. 绘制一个彩色三角形(使用for循环)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
for i in range(3):
turtle.color(colors[i % 6])
turtle.forward(100)
turtle.left(120)
```
19. 绘制一个彩色六边形(使用for循环)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
for i in range(6):
turtle.color(colors[i % 6])
turtle.forward(100)
turtle.right(60)
```
20. 绘制一个彩色圆形(使用for循环)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
for i in range(360):
turtle.color(colors[i % 6])
turtle.forward(1)
turtle.right(1)
```
21. 绘制一个彩色正方形(使用for循环)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
for i in range(4):
turtle.color(colors[i % 6])
turtle.forward(100)
turtle.right(90)
```
22. 绘制一个彩色正方形螺旋(使用while循环)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
i = 0
while i < 100:
turtle.color(colors[i % 6])
turtle.forward(i * 2)
turtle.right(90)
i += 1
```
23. 绘制一个彩色五角星(使用while循环)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
i = 0
while i < 5:
turtle.color(colors[i % 6])
turtle.forward(100)
turtle.right(144)
i += 1
```
24. 绘制一个彩色三角形(使用while循环)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
i = 0
while i < 3:
turtle.color(colors[i % 6])
turtle.forward(100)
turtle.left(120)
i += 1
```
25. 绘制一个彩色六边形(使用while循环)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
i = 0
while i < 6:
turtle.color(colors[i % 6])
turtle.forward(100)
turtle.right(60)
i += 1
```
26. 绘制一个彩色圆形(使用while循环)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
i = 0
while i < 360:
turtle.color(colors[i % 6])
turtle.forward(1)
turtle.right(1)
i += 1
```
27. 绘制一个彩色正方形(使用while循环)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
i = 0
while i < 4:
turtle.color(colors[i % 6])
turtle.forward(100)
turtle.right(90)
i += 1
```
28. 绘制一个正方形螺旋(使用函数)
```python
import turtle
def square_spiral():
for i in range(100):
turtle.forward(i * 2)
turtle.right(90)
square_spiral()
```
29. 绘制一个彩色正方形螺旋(使用函数)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
def color_square_spiral():
for i in range(100):
turtle.color(colors[i % 6])
turtle.forward(i * 2)
turtle.right(90)
color_square_spiral()
```
30. 绘制一个彩色五角星(使用函数)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
def color_star():
for i in range(5):
turtle.color(colors[i % 6])
turtle.forward(100)
turtle.right(144)
color_star()
```
31. 绘制一个彩色三角形(使用函数)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
def color_triangle():
for i in range(3):
turtle.color(colors[i % 6])
turtle.forward(100)
turtle.left(120)
color_triangle()
```
32. 绘制一个彩色六边形(使用函数)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
def color_hexagon():
for i in range(6):
turtle.color(colors[i % 6])
turtle.forward(100)
turtle.right(60)
color_hexagon()
```
33. 绘制一个彩色圆形(使用函数)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
def color_circle():
for i in range(360):
turtle.color(colors[i % 6])
turtle.forward(1)
turtle.right(1)
color_circle()
```
34. 绘制一个彩色正方形(使用函数)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
def color_square():
for i in range(4):
turtle.color(colors[i % 6])
turtle.forward(100)
turtle.right(90)
color_square()
```
35. 绘制一个彩色正方形螺旋(使用递归)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
def color_square_spiral_recursive(length):
if length > 0:
turtle.color(colors[length % 6])
turtle.forward(length * 2)
turtle.right(90)
color_square_spiral_recursive(length - 1)
color_square_spiral_recursive(99)
```
36. 绘制一个彩色五角星(使用递归)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
def color_star_recursive(length):
if length > 0:
turtle.color(colors[length % 6])
turtle.forward(length)
turtle.right(144)
color_star_recursive(length - 1)
color_star_recursive(100)
```
37. 绘制一个彩色三角形(使用递归)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
def color_triangle_recursive(length):
if length > 0:
turtle.color(colors[length % 6])
turtle.forward(length)
turtle.left(120)
color_triangle_recursive(length - 1)
color_triangle_recursive(100)
```
38. 绘制一个彩色六边形(使用递归)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
def color_hexagon_recursive(length):
if length > 0:
turtle.color(colors[length % 6])
turtle.forward(length)
turtle.right(60)
color_hexagon_recursive(length - 1)
color_hexagon_recursive(100)
```
39. 绘制一个彩色圆形(使用递归)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
def color_circle_recursive(length):
if length > 0:
turtle.color(colors[length % 6])
turtle.forward(1)
turtle.right(1)
color_circle_recursive(length - 1)
color_circle_recursive(360)
```
40. 绘制一个彩色正方形(使用递归)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
def color_square_recursive(length):
if length > 0:
turtle.color(colors[length % 6])
turtle.forward(length)
turtle.right(90)
color_square_recursive(length - 1)
color_square_recursive(100)
```
41. 绘制一个正方形螺旋(使用列表推导式)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
[length for length in range(0, 200, 2)
for color in [colors[length // 2 % 6]]
for _ in range(2)
for _ in [turtle.color(color), turtle.forward(length), turtle.right(90)]
]
```
42. 绘制一个彩色正方形螺旋(使用列表推导式)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
[(length, color) for length in range(0, 200, 2)
for color in [colors[length // 2 % 6]]
]
for length, color in _:
turtle.color(color)
turtle.forward(length)
turtle.right(90)
```
43. 绘制一个彩色五角星(使用列表推导式)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
[(100, colors[i % 6]) for i in range(5)]
for length, color in _:
turtle.color(color)
turtle.forward(length)
turtle.right(144)
```
44. 绘制一个彩色三角形(使用列表推导式)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
[(100, colors[i % 6]) for i in range(3)]
for length, color in _:
turtle.color(color)
turtle.forward(length)
turtle.left(120)
```
45. 绘制一个彩色六边形(使用列表推导式)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
[(100, colors[i % 6]) for i in range(6)]
for length, color in _:
turtle.color(color)
turtle.forward(length)
turtle.right(60)
```
46. 绘制一个彩色圆形(使用列表推导式)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
[(1, colors[i % 6]) for i in range(360)]
for length, color in _:
turtle.color(color)
turtle.forward(length)
turtle.right(1)
```
47. 绘制一个彩色正方形(使用列表推导式)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
[(100, colors[i % 6]) for i in range(4)]
for length, color in _:
turtle.color(color)
turtle.forward(length)
turtle.right(90)
```
48. 绘制一个正方形螺旋(使用lambda函数)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
square_spiral = lambda i: turtle.color(colors[i % 6]), turtle.forward(i * 2), turtle.right(90)
[square_spiral(i) for i in range(100)]
```
49. 绘制一个彩色正方形螺旋(使用lambda函数)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
color_square_spiral = lambda i: turtle.color(colors[i % 6]), turtle.forward(i * 2), turtle.right(90)
[color_square_spiral(i) for i in range(100)]
```
50. 绘制一个彩色五角星(使用lambda函数)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
color_star = lambda i: turtle.color(colors[i % 6]), turtle.forward(100), turtle.right(144)
[color_star(i) for i in range(5)]
```
51. 绘制一个彩色三角形(使用lambda函数)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
color_triangle = lambda i: turtle.color(colors[i % 6]), turtle.forward(100), turtle.left(120)
[color_triangle(i) for i in range(3)]
```
52. 绘制一个彩色六边形(使用lambda函数)
```python
import turtle
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
color_hexagon = lambda i: turtle.color(colors[i % 6]), turtle.forward(100), turtle.right(60)
[color_hex
阅读全文
相关推荐
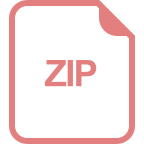















