禾木与海龟作图 题目描述 禾木最近自学了python中的海龟(turtle)作图,突发奇想,想用c++来实现。 二维平面上有一只海龟,一开始,海龟面向x轴正方向,位于坐标原点(0,0)。接下来海龟会接受一连串命令,每个命令可以用一个大写字母表示: 1. R表示海龟沿顺时针旋转90度; 2. L表示海龟沿逆时针旋转90度; 3. F表示海龟沿当前方向前进一个单元; 4. B表示海龟沿当前方向后退一个单元; 请你编写程序,求出结束时,海龟的位置坐标。
时间: 2023-06-08 10:08:10 浏览: 287
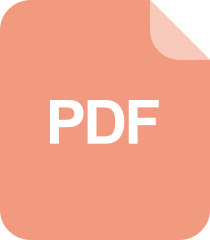
C++程序海龟图.pdf
ChitGPT: 好的,这是一道比较基础的编程题目。让我想一想,我需要设计一个海龟类,还需要一个方法来执行每一个命令。
首先,我定义一个Turtle类,有三个属性:x、y和direction。direction表示海龟当前面向的方向。
```
class Turtle:
def __init__(self):
self.x = 0
self.y = 0
self.direction = 'E'
```
其中,direction的初始值为'E',表示海龟最初面向x轴正方向。
接下来,我定义一个execute方法,用来执行命令。这里我采用了一个字典来存储每个命令对应的操作。可以看到,对于命令F和B,我需要根据当前方向来判断是沿x轴还是y轴前进或后退。
```
class Turtle:
def __init__(self):
self.x = 0
self.y = 0
self.direction = 'E'
def execute(self, commands):
for command in commands:
action = {
'R': self.turn_right,
'L': self.turn_left,
'F': self.forward,
'B': self.backward
}
action[command]()
def turn_right(self):
directions = ['N', 'E', 'S', 'W']
self.direction = directions[(directions.index(self.direction) + 1) % 4]
def turn_left(self):
directions = ['N', 'W', 'S', 'E']
self.direction = directions[(directions.index(self.direction) + 1) % 4]
def forward(self):
if self.direction == 'N':
self.y += 1
elif self.direction == 'E':
self.x += 1
elif self.direction == 'S':
self.y -= 1
else:
self.x -= 1
def backward(self):
if self.direction == 'N':
self.y -= 1
elif self.direction == 'E':
self.x -= 1
elif self.direction == 'S':
self.y += 1
else:
self.x += 1
```
最后,我写一个简单的测试代码来验证程序的正确性。
```
t = Turtle()
t.execute(['F', 'R', 'F', 'L', 'B'])
print(t.x, t.y)
```
这段代码表示海龟依次执行前进、右转、前进、左转、后退这几个命令。程序输出的坐标应该是(1, 1)。
阅读全文
相关推荐
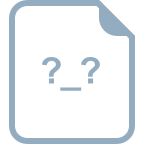
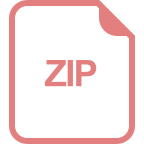
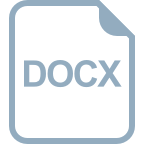
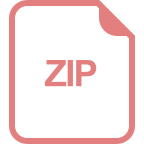
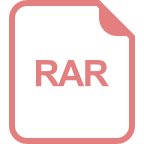
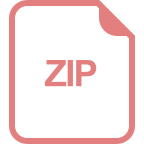
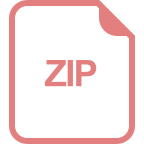
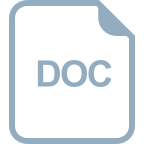
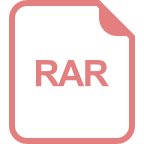
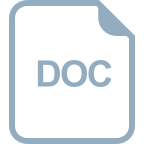
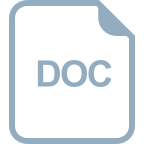





