数据结构,用队列逆置栈
时间: 2023-06-28 09:04:27 浏览: 117
可以使用两个队列来实现队列逆置栈的操作。具体步骤如下:
1. 将元素依次入队到队列1中;
2. 当队列1非空时,将队列1中除最后一个元素外的其他元素依次出队并入队到队列2中;
3. 将队列1中剩余的最后一个元素出队并作为栈顶元素;
4. 交换队列1和队列2的指针,使得队列1成为下一次入队的队列。
以下是实现该算法的 C++ 代码:
```cpp
#include <iostream>
#include <queue>
using namespace std;
class Stack {
public:
void push(int x) {
q1.push(x);
}
void pop() {
while (q1.size() > 1) {
q2.push(q1.front());
q1.pop();
}
q1.pop();
swap(q1, q2);
}
int top() {
while (q1.size() > 1) {
q2.push(q1.front());
q1.pop();
}
int top = q1.front();
q2.push(top);
q1.pop();
swap(q1, q2);
return top;
}
bool empty() {
return q1.empty() && q2.empty();
}
private:
queue<int> q1, q2;
};
int main() {
Stack s;
s.push(1);
s.push(2);
s.push(3);
cout << s.top() << endl; // 输出 3
s.pop();
cout << s.top() << endl; // 输出 2
s.push(4);
cout << s.top() << endl; // 输出 4
return 0;
}
```
在上述代码中,我们使用两个队列 `q1` 和 `q2` 来实现栈的操作。其中,`push` 操作直接将元素入队到队列1中;`pop` 操作则需要将队列1中除最后一个元素外的其他元素依次出队并入队到队列2中,最后再将队列1中剩余的最后一个元素出队;`top` 操作也需要将队列1中除最后一个元素外的其他元素依次出队并入队到队列2中,最后返回队列1中剩余的最后一个元素,同时将其入队到队列2中;`empty` 操作则需要同时判断队列1和队列2是否都为空。
阅读全文
相关推荐


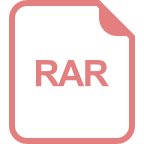




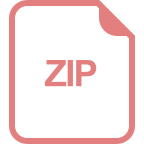
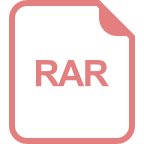








