用java写一个扫雷小程序
时间: 2023-12-29 14:27:38 浏览: 99
好的,我可以为您提供一个简单的Java扫雷小游戏程序,代码如下:
```java
import java.util.Random;
import java.util.Scanner;
public class Minesweeper {
static final int SIZE = 10; // 游戏区域大小
static final int MINES = 10; // 地雷数量
static int[][] board = new int[SIZE][SIZE]; // 游戏区域矩阵
static boolean[][] visible = new boolean[SIZE][SIZE]; // 是否可见
static boolean gameOver = false; // 游戏是否结束
public static void main(String[] args) {
initBoard();
printBoard();
Scanner input = new Scanner(System.in);
while (!gameOver) {
System.out.print("请输入坐标(x,y): ");
int x = input.nextInt();
int y = input.nextInt();
if (x < 0 || x >= SIZE || y < 0 || y >= SIZE) {
System.out.println("坐标不合法,请重新输入!");
} else if (visible[x][y]) {
System.out.println("该位置已经被揭示,请重新输入!");
} else {
gameOver = reveal(x, y);
printBoard();
}
}
System.out.println("游戏结束!");
}
// 初始化游戏区域
private static void initBoard() {
Random rand = new Random();
int count = 0;
while (count < MINES) {
int x = rand.nextInt(SIZE);
int y = rand.nextInt(SIZE);
if (board[x][y] != -1) {
board[x][y] = -1;
count++;
}
}
for (int i = 0; i < SIZE; i++) {
for (int j = 0; j < SIZE; j++) {
if (board[i][j] != -1) {
board[i][j] = countMines(i, j);
}
}
}
}
// 统计周围地雷数量
private static int countMines(int x, int y) {
int count = 0;
for (int i = x - 1; i <= x + 1; i++) {
for (int j = y - 1; j <= y + 1; j++) {
if (i >= 0 && i < SIZE && j >= 0 && j < SIZE && board[i][j] == -1) {
count++;
}
}
}
return count;
}
// 揭示某个位置
private static boolean reveal(int x, int y) {
visible[x][y] = true;
if (board[x][y] == -1) {
System.out.println("你踩到地雷了!");
return true;
} else if (board[x][y] == 0) {
for (int i = x - 1; i <= x + 1; i++) {
for (int j = y - 1; j <= y + 1; j++) {
if (i >= 0 && i < SIZE && j >= 0 && j < SIZE && !visible[i][j]) {
reveal(i, j);
}
}
}
}
return checkWin();
}
// 检查是否胜利
private static boolean checkWin() {
for (int i = 0; i < SIZE; i++) {
for (int j = 0; j < SIZE; j++) {
if (!visible[i][j] && board[i][j] != -1) {
return false;
}
}
}
System.out.println("你赢了!");
return true;
}
// 打印游戏区域
private static void printBoard() {
System.out.print(" ");
for (int i = 0; i < SIZE; i++) {
System.out.print(i + " ");
}
System.out.println();
for (int i = 0; i < SIZE; i++) {
System.out.print(i + " ");
for (int j = 0; j < SIZE; j++) {
if (visible[i][j]) {
if (board[i][j] == -1) {
System.out.print("* ");
} else {
System.out.print(board[i][j] + " ");
}
} else {
System.out.print(". ");
}
}
System.out.println();
}
}
}
```
这个程序实现了一个简单的10x10的扫雷游戏,其中有10个地雷。玩家通过输入坐标来揭示游戏区域中的某个位置,游戏会根据该位置周围地雷的数量进行相应的处理,直到游戏结束。
阅读全文
相关推荐





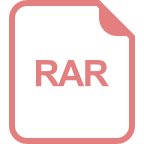
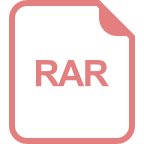

