扫雷小游戏java代码
时间: 2024-01-03 10:01:55 浏览: 30
下面是一个简单的扫雷小游戏的Java代码示例:
```java
import java.util.*;
public class Minesweeper {
private static final int SIZE = 10;
private static final int MINE_COUNT = 10;
private static final char UNKNOWN = '-';
private static final char MINE = '*';
private static final char EXPLORED = ' ';
public static void main(String[] args) {
char[][] board = initializeBoard();
placeMines(board);
playGame(board);
}
public static char[][] initializeBoard() {
char[][] board = new char[SIZE][SIZE];
for (char[] row : board) {
Arrays.fill(row, UNKNOWN);
}
return board;
}
public static void placeMines(char[][] board) {
Random rand = new Random();
int count = 0;
while (count < MINE_COUNT) {
int row = rand.nextInt(SIZE);
int col = rand.nextInt(SIZE);
if (board[row][col] != MINE) {
board[row][col] = MINE;
count++;
}
}
}
public static void playGame(char[][] board) {
Scanner scanner = new Scanner(System.in);
int exploredCount = 0;
while (exploredCount < SIZE * SIZE - MINE_COUNT) {
printBoard(board);
System.out.print("请输入要探索的行坐标:");
int row = scanner.nextInt();
System.out.print("请输入要探索的列坐标:");
int col = scanner.nextInt();
if (board[row][col] == MINE) {
System.out.println("你被炸死了!");
break;
}
explore(board, row, col);
exploredCount++;
}
if (exploredCount == SIZE * SIZE - MINE_COUNT) {
System.out.println("恭喜你,你赢了!");
}
}
public static void explore(char[][] board, int row, int col) {
if (board[row][col] != UNKNOWN) {
return;
}
int count = 0;
for (int i = -1; i <= 1; i++) {
for (int j = -1; j <= 1; j++) {
if (isValidCell(row + i, col + j) && board[row + i][col + j] == MINE) {
count++;
}
}
}
if (count == 0) {
board[row][col] = EXPLORED;
for (int i = -1; i <= 1; i++) {
for (int j = -1; j <= 1; j++) {
if (isValidCell(row + i, col + j)) {
explore(board, row + i, col + j);
}
}
}
} else {
board[row][col] = (char) (count + '0');
}
}
public static boolean isValidCell(int row, int col) {
return (row >= 0 && row < SIZE && col >= 0 && col < SIZE);
}
public static void printBoard(char[][] board) {
System.out.println("当前棋盘状态:");
for (char[] row : board) {
for (char cell : row) {
System.out.print(cell + " ");
}
System.out.println();
}
}
}
```
这个游戏使用字符数组表示游戏棋盘,其中未探索的单元格用"-"表示,雷的位置用"*"表示,已探索的单元格用空格表示,数字表示周围有多少个雷。游戏开始时,先生成一个大小为10x10的棋盘,并在随机位置放置10个雷。然后玩家依次输入要探索的行坐标和列坐标,程序会判断该位置是否有雷,如果有就游戏结束,否则会根据该位置周围雷的数量进行递归探索。如果玩家成功找出所有的非雷单元格,那么游戏胜利。
相关推荐
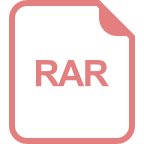














