扫雷小游戏Java代码详细版
时间: 2024-09-21 07:05:11 浏览: 34
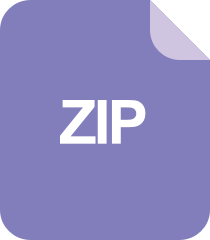
Java做的扫雷小游戏.zip
扫雷游戏是一个经典的计算机博弈游戏,通常涉及到网格中的数字表示周围地雷的数量。下面是一个简单的Java版本的扫雷游戏代码框架示例:
```java
import java.util.Random;
public class Minesweeper {
private int[][] board; // 游戏矩阵
private int width, height; // 网格尺寸
private int mines; // 地雷总数
public Minesweeper(int rows, int cols) {
this.width = cols;
this.height = rows;
mines = (int) Math.ceil((double) rows * cols / 10); // 一般假设每10格设一颗地雷
initializeBoard();
placeMines();
}
// 初始化空的grid
private void initializeBoard() {
board = new int[height][width];
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
board[i][j] = 0;
}
}
}
// 随机放置地雷
private void placeMines() {
Random random = new Random();
for (int mine = 0; mine < mines; mine++) {
int row = random.nextInt(height);
int col = random.nextInt(width);
if (board[row][col] == 0) { // 只有未标记的位置才能放置
board[row][col] = -1;
} else {
placeMinesRecursively(row, col);
}
}
}
// 递归地雷放置
private void placeMinesRecursively(int row, int col) {
// 如果当前位置已有地雷或越界,返回
if (row < 0 || row >= height || col < 0 || col >= width || board[row][col] != 0) return;
// 标记当前位置为地雷
board[row][col] = -1;
// 检查周围的8个邻居是否需要放置地雷
checkNeighbors(row, col);
}
// 检查并计算邻居数
private void checkNeighbors(int row, int col) {
int count = 0;
for (int neighborRow = row - 1; neighborRow <= row + 1; neighborRow++) {
for (int neighborCol = col - 1; neighborCol <= col + 1; neighborCol++) {
if (neighborRow != row || neighborCol != col && board[neighborRow][neighborCol] == -1) {
count++;
}
}
}
board[row][col] += count; // 更新当前位置的值,代表周围地雷数
}
// 用户点击事件处理,这里只是一个简化版本
public void click(int row, int col) {
// ... 实现逻辑,如检查是否有地雷、显示数字等
}
// 主函数,用于启动游戏
public static void main(String[] args) {
Minesweeper game = new Minesweeper(10, 10);
// 游戏循环...
}
}
```
这只是一个基础的框架,实际的游戏会更复杂,包括用户界面交互、游戏结束条件判断以及错误处理等。如果你对这个代码感兴趣,可以在此基础上添加更多功能。
阅读全文
相关推荐
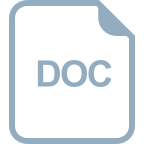
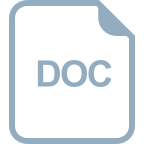

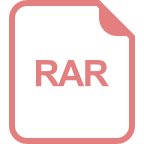
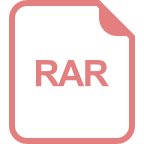
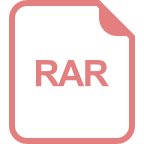
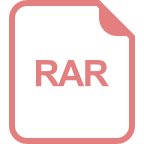
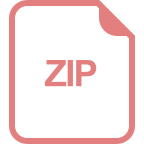
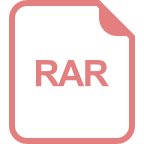
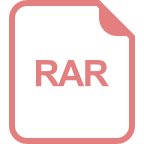





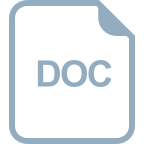