用c++ 用指针编写程序,当从键盘输入一个字符串后要求: (1)统计这个字符串的长度(用子函数,参数是字符指针,不能用 strlen) (2)统计这个字符串中数字字符的个数(用子函数,参数是宇符指针) (3)能够将这个字符串的所有英文字符转换为对应的大写字母。(用一个 子函数,参数是字符指针)
时间: 2024-02-27 18:55:26 浏览: 24
好的,这是一个比较基础的指针编程题目,下面是代码实现:
```c++
#include <iostream>
using namespace std;
// 统计字符串长度
int getLength(char* str) {
int len = 0;
while (*str++ != '\0') {
len++;
}
return len;
}
// 统计数字字符个数
int getDigitCount(char* str) {
int count = 0;
while (*str != '\0') {
if (*str >= '0' && *str <= '9') {
count++;
}
str++;
}
return count;
}
// 将英文字符转换为大写字母
void toUpper(char* str) {
while (*str != '\0') {
if (*str >= 'a' && *str <= 'z') {
*str -= 32;
}
str++;
}
}
int main() {
char str[100];
cout << "请输入一个字符串: ";
cin >> str;
// 统计字符串长度
int len = getLength(str);
cout << "字符串长度为: " << len << endl;
// 统计数字字符个数
int digitCount = getDigitCount(str);
cout << "数字字符个数为: " << digitCount << endl;
// 将英文字符转换为大写字母
toUpper(str);
cout << "转换后的字符串为: " << str << endl;
return 0;
}
```
这个程序基本上实现了题目要求,你可以在本地编译运行一下看看。需要注意的是,这个程序没有进行输入字符串长度的判断,如果输入的字符串长度超过了数组长度,就会导致未知错误。在实际的开发中,需要对输入进行判断并进行相应的处理。
相关推荐
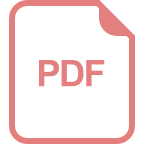














