yolo v5 txt检测框显示代码
时间: 2023-09-14 10:07:13 浏览: 55
以下是使用YOLOv5检测文本,并在检测结果上绘制检测框的代码示例:
```python
import cv2
import torch
import numpy as np
from models.experimental import attempt_load
from utils.general import non_max_suppression
# 加载YOLOv5模型
weights = 'path/to/weights.pt'
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
model = attempt_load(weights, map_location=device)
# 定义类别名称和颜色
class_names = ['text']
colors = [[255, 0, 0]]
# 加载测试图片
img_path = 'path/to/image.jpg'
img = cv2.imread(img_path)
# 图片预处理
img_size = 640
img = cv2.resize(img, (img_size, img_size))
img = img[:, :, ::-1].transpose(2, 0, 1)
img = np.ascontiguousarray(img)
img = torch.from_numpy(img).to(device)
# 模型推理
model.eval()
with torch.no_grad():
pred = model(img.unsqueeze(0))[0]
pred = non_max_suppression(pred, conf_thres=0.5, iou_thres=0.5)
# 绘制检测框
if pred[0] is not None:
pred = pred[0]
for det in pred:
x1, y1, x2, y2, conf, cls = det
x1, y1, x2, y2 = int(x1), int(y1), int(x2), int(y2)
label = f'{class_names[int(cls)]} {conf:.2f}'
color = colors[int(cls)]
cv2.rectangle(img, (x1, y1), (x2, y2), color, 2)
cv2.putText(img, label, (x1, y1 - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, color, 2)
# 显示结果
cv2.imshow('result', img[:, :, ::-1])
cv2.waitKey(0)
```
其中,`attempt_load`函数用于加载YOLOv5模型,`non_max_suppression`函数用于进行非极大值抑制,过滤重叠的检测结果。使用`cv2.rectangle`和`cv2.putText`函数在检测结果上绘制检测框和标签,最后使用`cv2.imshow`函数显示结果。
相关推荐
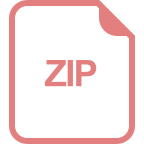
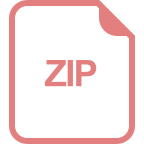














