if (!(ofmt_ctx->oformat->flags & AVFMT_NOFILE))
时间: 2024-06-05 15:09:25 浏览: 233
这段代码判断输出格式是否需要打开文件,如果需要则执行if语句中的代码,否则跳过if语句。具体来说,AVFMT_NOFILE是一个标志位,表示该输出格式不需要打开文件。因此,如果该标志位没有被设置,则需要打开文件,否则不需要。ofmt_ctx是一个输出格式上下文结构体,包含了输出格式相关的信息,包括是否需要打开文件。
相关问题
Nal 数据通过av_interleaved_write_frame 输出ts格式
将 NAL 数据输出到 TS 格式文件中,可以按照以下步骤进行:
1. 初始化 AVFormatContext 和 AVOutputFormat,设置输出文件名和格式参数。
```c
AVFormatContext *ofmt_ctx = NULL;
avformat_alloc_output_context2(&ofmt_ctx, NULL, "mpegts", out_filename);
AVOutputFormat *ofmt = ofmt_ctx->oformat;
```
2. 打开输出文件。
```c
if (!(ofmt->flags & AVFMT_NOFILE)) {
if (avio_open(&ofmt_ctx->pb, out_filename, AVIO_FLAG_WRITE) < 0) {
printf("Could not open output file '%s'", out_filename);
return -1;
}
}
```
3. 添加视频流到输出文件中,设置编码参数,打开编码器。
```c
AVStream *out_stream = avformat_new_stream(ofmt_ctx, NULL);
AVCodecParameters *codecpar = out_stream->codecpar;
codecpar->codec_id = AV_CODEC_ID_H264;
codecpar->codec_type = AVMEDIA_TYPE_VIDEO;
codecpar->format = AV_PIX_FMT_YUV420P;
codecpar->width = width;
codecpar->height = height;
codecpar->extradata_size = sps_len + pps_len;
codecpar->extradata = av_mallocz(sps_len + pps_len + AV_INPUT_BUFFER_PADDING_SIZE);
memcpy(codecpar->extradata, sps_pps_data, sps_len + pps_len);
out_stream->time_base = (AVRational){1, fps};
AVCodec *codec = avcodec_find_encoder(codecpar->codec_id);
AVCodecContext *codec_ctx = avcodec_alloc_context3(codec);
avcodec_parameters_to_context(codec_ctx, codecpar);
codec_ctx->time_base = out_stream->time_base;
codec_ctx->gop_size = 10;
codec_ctx->max_b_frames = 1;
codec_ctx->bit_rate = bitrate;
codec_ctx->rc_min_rate = bitrate;
codec_ctx->rc_max_rate = bitrate;
codec_ctx->rc_buffer_size = bitrate;
avcodec_open2(codec_ctx, codec, NULL);
```
4. 循环读取 NAL 数据,将数据写入 AVPacket 中,并设置时间戳和时长。
```c
while (read_len > 0) {
AVPacket pkt;
av_init_packet(&pkt);
pkt.data = buf;
pkt.size = read_len;
pkt.pts = pts++;
pkt.dts = pkt.pts;
pkt.duration = 1;
pkt.stream_index = out_stream->index;
av_interleaved_write_frame(ofmt_ctx, &pkt);
av_packet_unref(&pkt);
read_len = fread(buf, 1, buf_size, fp);
}
```
5. 写入文件尾,并释放资源。
```c
av_write_trailer(ofmt_ctx);
avcodec_close(codec_ctx);
avcodec_free_context(&codec_ctx);
av_freep(&codecpar->extradata);
avio_close(ofmt_ctx->pb);
avformat_free_context(ofmt_ctx);
```
完整代码示例可以参考以下链接:https://gist.github.com/mstorsjo/012b5861e1fca20f1b2b961d853e49ec
Error: Quartus Prime Analysis & Synthesis was unsuccessful. 3 errors, 20 warnings Error: Peak virtual memory: 4801 megabytes Error: Processing ended: Fri Jun 02 14:51:24 2023 Error: Elapsed time: 00:00:20 Error: Total CPU time (on all processors): 00:00:36
->index;
ret = av_interleaved_write_frame(ofmt_ctx, &pkt);
if (ret < 0) {
这个错误提示意味着 Quartus Prime 分析和综合过程中存在 3 个错误和 20 个 std::cerr << "Failed to write packet to output context" << std::endl;
return -1;
}
av_packet警告。其中最后一行提示了处理结束的时间和总共使用的 CPU 时间。
这种错误通常是由_unref(&pkt);
}
}
av_frame_free(&input_frame);
}
av_packet_unref(&pkt);
}
设计中存在语法错误、逻辑错误或约束问题等引起的。您可以查看 Quartus Prime 提供的 // Write the output trailer
av_write_trailer(ofmt_ctx);
// Free resources
avcodec_free_context(&video_codec详细报告,以了解具体的错误和警告信息,并根据报告中的建议进行更改。_ctx);
av_frame_free(&video_frame);
avformat_close_input(&ifmt_ctx);
if (ofmt_ctx && !(of在报告中,错误和警告通常会有详细的描述和定位信息,以便您快速找到mt_ctx->oformat->flags & AVFMT_NOFILE)) {
avio_closep(&ofmt_ctx->pb);
}
并解决问题。
此外,您还可以检查设计中是否存在缺失的文件或库,或者是否存在版本 avformat_free_context(ofmt_ctx);
return 0;
}
```
需要注意的是,这只是一个简单的不匹配的问题。如果您无法解决问题,请提供更多上下文信息,例如报告截图或示例,实际使用时还需要考虑很多细节问题,例如错误处理、内存管理、流控制等。
阅读全文
相关推荐
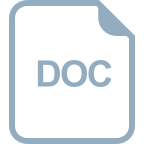
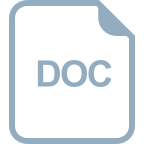
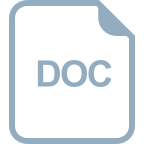
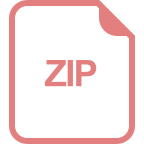
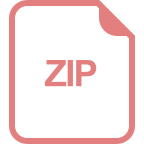
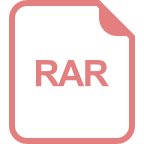
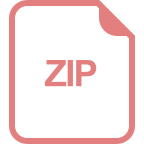
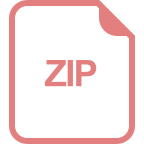
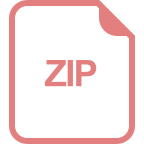
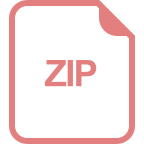
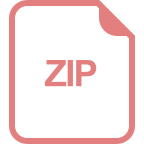