c++指定路径遍历文件
时间: 2023-11-29 19:05:18 浏览: 111
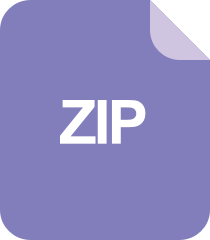
c++遍历文件夹
要遍历指定路径下的文件,可以使用Python的os模块。具体步骤如下:
1. 导入os模块:
```python
import os
```
2. 使用os模块中的listdir()函数返回指定路径下的所有文件和文件夹:
```python
file_list = os.listdir('/path/to/directory')
```
3. 遍历文件列表,判断每个元素是文件还是文件夹,并进行相应的操作:
```python
for file in file_list:
file_path = os.path.join('/path/to/directory', file) # 获取文件路径
if os.path.isfile(file_path): # 判断是否为文件
# 对文件进行操作
elif os.path.isdir(file_path): # 判断是否为文件夹
# 对文件夹进行操作
```
其中,os.path.join()函数用于拼接路径,避免因为路径分隔符不同导致的错误。具体使用方法可以参考Python官方文档。
阅读全文
相关推荐
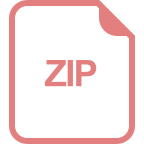
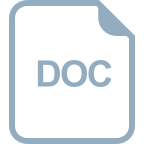
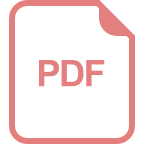
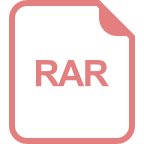
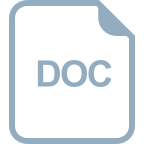
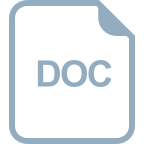
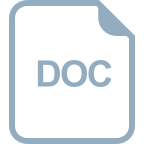









