写一个Python数据自动录入MySQL云服务器的代码
时间: 2023-09-18 10:06:02 浏览: 158
### 回答1:
答:以下是将数据自动录入MySQL云服务器的Python代码:import pymysql# Connect to the database
connection = pymysql.connect(host='localhost',
user='user',
password='passwd',
db='database',
charset='utf8mb4',
cursorclass=pymysql.cursors.DictCursor)try:
with connection.cursor() as cursor:
# Create a new record
sql = "INSERT INTO `table` (`column1`, `column2`, `column3`) VALUES (%s, %s, %s)"
cursor.execute(sql, ('value1', 'value2', 'value3')) # connection is not autocommit by default. So you must commit to save
# your changes.
connection.commit()finally:
connection.close()
### 回答2:
使用Python实现数据自动录入MySQL云服务器的代码如下:
```python
import pymysql
def insert_data_to_mysql(data):
# 连接MySQL数据库
conn = pymysql.connect(
host='云服务器IP地址',
port=3306,
user='数据库用户名',
password='数据库密码',
database='数据库名',
charset='utf8'
)
# 创建游标对象
cursor = conn.cursor()
try:
# 执行插入操作
for d in data:
sql = "INSERT INTO 表名(字段1, 字段2, 字段3) VALUES (%s, %s, %s)"
cursor.execute(sql, (d['字段1'], d['字段2'], d['字段3']))
# 提交事务
conn.commit()
print("数据插入成功")
except Exception as e:
# 出现异常时回滚
conn.rollback()
print("数据插入失败:" + str(e))
finally:
# 关闭游标和连接
cursor.close()
conn.close()
# 测试代码
data = [
{'字段1': '值1', '字段2': '值2', '字段3': '值3'},
{'字段1': '值4', '字段2': '值5', '字段3': '值6'}
]
insert_data_to_mysql(data)
```
以上代码通过pymysql库实现了与MySQL数据库的连接和数据插入操作。其中,需要根据实际情况填写云服务器的IP地址、数据库用户名、密码、数据库名、表名,以及对应的字段和要插入的数据。在测试代码中,data为要插入的数据,可以根据实际需求进行修改。
### 回答3:
要实现Python数据自动录入MySQL云服务器的代码,可以使用Python中的MySQL Connector库进行操作。下面是一个简单的示例代码:
```python
import mysql.connector
# 连接到MySQL服务器
cnx = mysql.connector.connect(
host='云服务器地址',
user='数据库用户名',
password='数据库密码',
database='数据库名称'
)
# 创建游标对象
cursor = cnx.cursor()
# 定义插入数据的SQL语句
insert_stmt = "INSERT INTO 表名 (字段1, 字段2, 字段3) VALUES (%s, %s, %s)"
# 定义要插入的数据
data = [
('数据1', '数据2', '数据3'),
('数据4', '数据5', '数据6'),
('数据7', '数据8', '数据9')
]
try:
# 执行插入数据的SQL语句
cursor.executemany(insert_stmt, data)
# 提交事务
cnx.commit()
print("数据插入成功!")
except mysql.connector.Error as err:
# 发生错误时回滚事务
cnx.rollback()
print("数据插入失败:{}".format(err))
# 关闭游标和数据库连接
cursor.close()
cnx.close()
```
在代码中,需要替换`云服务器地址`、`数据库用户名`、`数据库密码`、`数据库名称`以及`表名`、`字段1`、`字段2`、`字段3`为实际的值。
以上代码连接到MySQL服务器,并通过`executemany`方法批量插入数据。如果插入成功,则提交事务;如果发生错误,则回滚事务。在最后要记得关闭游标和数据库连接。
请注意,在使用该代码前需要确保已经安装了MySQL Connector库,可以使用`pip install mysql-connector-python`进行安装。
阅读全文
相关推荐
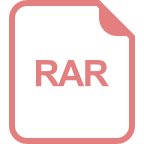
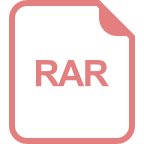
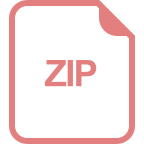
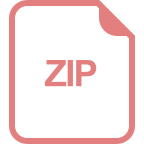
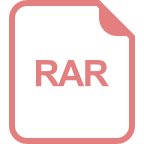
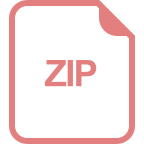
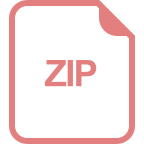
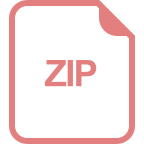
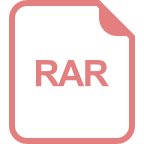
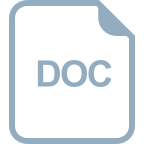
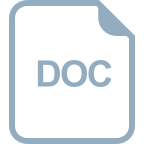
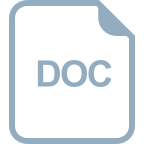
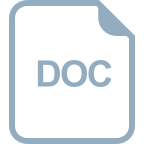
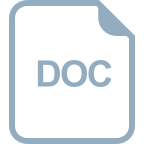
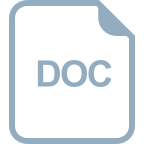
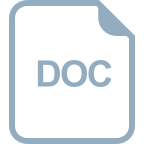


