在实现请求转发器和参数自动校验功能的前提下,参数校验的方式要做成可配置的 定义一个接口,接口中定义一个validate方法,使得在每个注解(如@NotNull等)中可以 配置校验参数时使用的此接口的实现类 应有一个默认的参数校验器生成完整的代码例子,手写实现
时间: 2024-05-16 17:15:53 浏览: 10
首先,我们需要定义一个参数校验器的接口,如下所示:
```java
public interface ParamValidator {
void validate(Object param) throws ParamValidateException;
}
```
然后,我们需要在每个注解中添加一个参数,用于指定校验参数时使用的实现类,如下所示:
```java
public @interface NotNull {
String message() default "参数不能为空";
Class<? extends ParamValidator> validator() default DefaultParamValidator.class;
}
```
在上面的注解中,我们添加了一个validator属性,默认值为DefaultParamValidator.class,表示如果没有特别指定校验器,则使用默认的校验器。
接下来,我们需要实现一个默认的校验器,如下所示:
```java
public class DefaultParamValidator implements ParamValidator {
@Override
public void validate(Object param) throws ParamValidateException {
if (param == null) {
throw new ParamValidateException("参数不能为空");
}
}
}
```
在上面的默认校验器中,我们只是简单地判断参数是否为null,如果是null,则抛出ParamValidateException异常。
最后,我们需要实现一个请求转发器,它的作用是对每个接口方法的参数进行校验,如下所示:
```java
public class RequestDispatcher {
public static Object dispatch(Object obj, Method method, Object[] args) throws Throwable {
Parameter[] parameters = method.getParameters();
if (parameters == null || parameters.length == 0) {
return method.invoke(obj, args);
} else {
for (int i = 0; i < parameters.length; i++) {
Parameter parameter = parameters[i];
Object arg = args[i];
Annotation[] annotations = parameter.getAnnotations();
for (Annotation annotation : annotations) {
Class<? extends Annotation> type = annotation.annotationType();
Method method1 = type.getDeclaredMethod("validator");
Class<? extends ParamValidator> validatorClass = (Class<? extends ParamValidator>) method1.invoke(annotation);
ParamValidator validator = validatorClass.newInstance();
validator.validate(arg);
}
}
return method.invoke(obj, args);
}
}
}
```
在上面的请求转发器中,我们首先获取了接口方法的所有参数,然后对每个参数进行校验。对于每个参数,我们获取它的所有注解,并通过反射获取注解中配置的校验器类,然后实例化校验器并调用validate方法对参数进行校验。如果参数校验不通过,则抛出ParamValidateException异常。如果所有参数校验通过,则调用接口方法并返回结果。
最后,我们可以通过下面的代码使用请求转发器:
```java
public interface DemoService {
void test(@NotNull String name);
}
public class DemoServiceImpl implements DemoService {
@Override
public void test(String name) {
System.out.println("Hello " + name);
}
}
public class Main {
public static void main(String[] args) throws Throwable {
DemoService demoService = new DemoServiceImpl();
Method method = demoService.getClass().getMethod("test", String.class);
Object[] params = new Object[]{"world"};
RequestDispatcher.dispatch(demoService, method, params);
}
}
```
在上面的代码中,我们定义了一个DemoService接口,它有一个test方法,其中name参数使用了@NotNull注解。我们实现了一个DemoServiceImpl类来实现该接口。然后,我们使用RequestDispatcher.dispatch方法来调用test方法,并传入参数。如果参数校验不通过,则会抛出ParamValidateException异常。如果参数校验通过,则会输出"Hello world"。
相关推荐
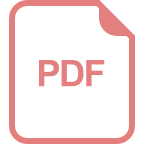
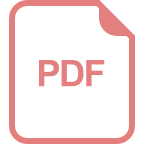















