1. 键盘输入10个学生的成绩,试编制一个程序统计60~69分,70~79分,80-89分,90~99分及100分的人数,分别存放到S6、S7、S8、S9及S10单元中。
时间: 2023-09-18 08:06:02 浏览: 80
### 回答1:
下面是一个简单的实现这个功能的Python代码:
```python
# 初始化计数器
s6 = s7 = s8 = s9 = s10 = 0
# 循环输入学生成绩,并统计人数
for i in range(10):
score = int(input("请输入第{}位学生的成绩:".format(i+1)))
if score == 100:
s10 += 1
elif score >= 90:
s9 += 1
elif score >= 80:
s8 += 1
elif score >= 70:
s7 += 1
elif score >= 60:
s6 += 1
# 输出统计结果
print("60~69分的人数为:", s6)
print("70~79分的人数为:", s7)
print("80~89分的人数为:", s8)
print("90~99分的人数为:", s9)
print("100分的人数为:", s10)
```
运行程序后,会依次提示输入每个学生的成绩,输入完成后会输出各个分数段的人数统计结果。
### 回答2:
可以使用数组和循环来编写程序,实现对10个学生的成绩进行统计。
首先声明一个长度为11的整型数组,用来存放成绩统计结果,数组下标代表分数段。
然后使用循环,循环10次,每次循环读取一个学生的成绩并将其存入数组对应的分数段中。
最后,将数组中的统计结果输出到对应的S6、S7、S8、S9及S10单元中。
以下是一个示例代码:
```python
def count_scores():
scores = [0] * 11 # 初始化一个长度为11的数组,初始值全为0
for i in range(10):
score = int(input("请输入第%d个学生的成绩:" % (i + 1)))
scores[score // 10] += 1 # 根据学生的成绩将对应的数组元素加1
results = []
for i in range(6, 11):
results.append("S%d: %d人" % (i, scores[i]))
return results
statistics = count_scores()
for result in statistics:
print(result)
```
运行上述代码,依次输入10个学生的成绩,程序会统计并将结果输出。其中,S6表示60~69分的人数,S7表示70~79分的人数,S8表示80~89分的人数,S9表示90~99分的人数,S10表示100分的人数。
### 回答3:
首先,我们可以使用数组来存储学生的成绩,数组的长度为10,用来存储10个学生的成绩。
然后,我们使用循环来逐个获取学生的成绩并进行统计,循环的次数为10次。
在循环中,我们使用if语句来判断学生的成绩属于哪个分数段,并进行相应的统计。统计每个分数段的人数可以用一个整型变量来进行累加。
最后,将统计得到的人数分别存放到S6、S7、S8、S9及S10单元中,可以通过给数组的相应元素赋值的方式实现。
以下是一个可能的解决方案:
```python
scores = [] # 存储学生的成绩的数组
# 输入学生的成绩
for i in range(10):
score = int(input("请输入第{}个学生的成绩:".format(i+1)))
scores.append(score)
# 初始化分数段的人数
count_s6 = 0 # 60-69分的人数
count_s7 = 0 # 70-79分的人数
count_s8 = 0 # 80-89分的人数
count_s9 = 0 # 90-99分的人数
count_s10 = 0 # 100分的人数
# 统计分数段的人数
for score in scores:
if score >= 60 and score <= 69:
count_s6 += 1
elif score >= 70 and score <= 79:
count_s7 += 1
elif score >= 80 and score <= 89:
count_s8 += 1
elif score >= 90 and score <= 99:
count_s9 += 1
elif score == 100:
count_s10 += 1
# 存放统计结果到数组中
result = [0, 0, 0, 0, 0]
result[0] = count_s6
result[1] = count_s7
result[2] = count_s8
result[3] = count_s9
result[4] = count_s10
print("60-69分的人数:{}".format(result[0]))
print("70-79分的人数:{}".format(result[1]))
print("80-89分的人数:{}".format(result[2]))
print("90-99分的人数:{}".format(result[3]))
print("100分的人数:{}".format(result[4]))
```
这样,我们就可以得到每个分数段的人数,并将结果存放到相应的单元中。
相关推荐
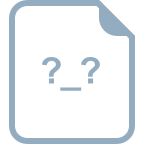
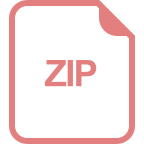
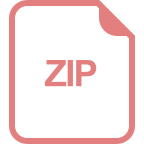
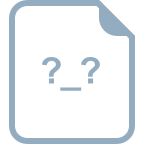
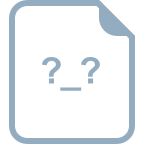
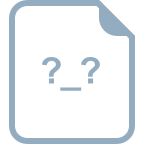
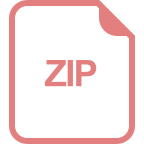
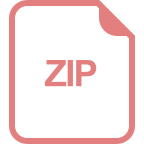
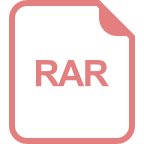
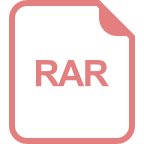
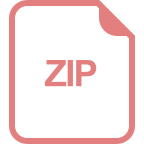
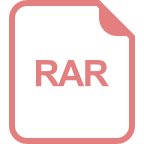
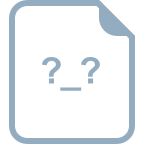