completablefuture.runAsync设定返回值
时间: 2023-11-07 14:20:37 浏览: 41
CompletableFuture.runAsync() 方法返回的是一个 CompletableFuture<Void>,也就是说该方法没有返回值。它表示一个异步执行的计算,当计算完成时,可以执行一些操作,但是不能通过它来获取计算结果。如果需要获取计算结果,则可以使用带返回值的 CompletableFuture.supplyAsync() 方法。
相关问题
CompletableFuture.runAsync 使用样例
CompletableFuture.runAsync是Java 8中的一个异步执行任务的方法。它可以在后台线程中执行一个Runnable任务,并返回一个CompletableFuture对象,该对象可以用于获取任务的执行结果或者进行后续的操作。
下面是一个使用CompletableFuture.runAsync的样例:
```java
import java.util.concurrent.CompletableFuture;
public class CompletableFutureExample {
public static void main(String[] args) {
// 创建一个CompletableFuture对象,执行一个简单的任务
CompletableFuture<Void> future = CompletableFuture.runAsync(() -> {
// 在后台线程中执行的任务
System.out.println("Task is running in background thread");
// 模拟任务执行时间
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("Task completed");
});
// 主线程可以继续执行其他操作
// 等待任务完成并获取结果
future.join();
System.out.println("Main thread continues to execute");
}
}
```
上述代码中,我们创建了一个CompletableFuture对象,并使用runAsync方法执行一个简单的任务。任务会在后台线程中执行,打印一些信息,并模拟任务执行时间。主线程在任务执行期间可以继续执行其他操作。最后,我们使用join方法等待任务完成,并打印一条信息。
CompletableFuture.runAsync使用距离
CompletableFuture.runAsync是Java 8中的一个异步执行任务方法,它可以在后台线程中执行指定的任务,而不会阻塞主线程。下面是一个使用CompletableFuture.runAsync的示例[^1]:
```java
CompletableFuture<Void> future = CompletableFuture.runAsync(() -> {
// 执行需要在后台线程中执行的任务
System.out.println("Hello, world!");
});
// 等待任务执行完成
future.get();
```
在这个示例中,我们使用CompletableFuture.runAsync方法创建了一个CompletableFuture对象,该对象会在后台线程中执行一个简单的任务,即打印"Hello, world!"。然后,我们使用future.get()方法等待任务执行完成。
需要注意的是,CompletableFuture.runAsync方法返回的是一个CompletableFuture<Void>对象,表示任务执行完成后不返回任何结果。
相关推荐
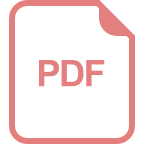
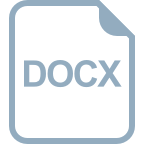
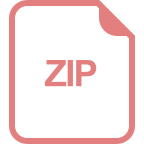












