java大作业聊天系统数据库设计代码
时间: 2023-07-29 08:07:06 浏览: 54
以下是一个简单的聊天系统数据库设计代码,使用Java编写:
```java
import java.sql.*;
public class ChatSystemDatabase {
private Connection conn;
public ChatSystemDatabase(String dbUrl, String username, String password) {
try {
conn = DriverManager.getConnection(dbUrl, username, password);
} catch (SQLException e) {
System.out.println("Error connecting to database: " + e.getMessage());
}
}
public void createTables() {
try {
Statement stmt = conn.createStatement();
String userTable = "CREATE TABLE users (id INT PRIMARY KEY, username VARCHAR(50), password VARCHAR(50))";
String messageTable = "CREATE TABLE messages (id INT PRIMARY KEY, senderId INT, receiverId INT, content VARCHAR(500), timestamp TIMESTAMP)";
stmt.executeUpdate(userTable);
stmt.executeUpdate(messageTable);
System.out.println("Tables created successfully!");
} catch (SQLException e) {
System.out.println("Error creating tables: " + e.getMessage());
}
}
public void addUser(int id, String username, String password) {
try {
String query = "INSERT INTO users (id, username, password) VALUES (?, ?, ?)";
PreparedStatement pstmt = conn.prepareStatement(query);
pstmt.setInt(1, id);
pstmt.setString(2, username);
pstmt.setString(3, password);
pstmt.executeUpdate();
System.out.println("User added successfully!");
} catch (SQLException e) {
System.out.println("Error adding user: " + e.getMessage());
}
}
public void addMessage(int id, int senderId, int receiverId, String content, Timestamp timestamp) {
try {
String query = "INSERT INTO messages (id, senderId, receiverId, content, timestamp) VALUES (?, ?, ?, ?, ?)";
PreparedStatement pstmt = conn.prepareStatement(query);
pstmt.setInt(1, id);
pstmt.setInt(2, senderId);
pstmt.setInt(3, receiverId);
pstmt.setString(4, content);
pstmt.setTimestamp(5, timestamp);
pstmt.executeUpdate();
System.out.println("Message added successfully!");
} catch (SQLException e) {
System.out.println("Error adding message: " + e.getMessage());
}
}
public void closeConnection() {
try {
conn.close();
System.out.println("Database connection closed successfully!");
} catch (SQLException e) {
System.out.println("Error closing database connection: " + e.getMessage());
}
}
}
```
这个数据库设计包括两个表:一个存储用户信息,另一个存储消息信息。用户表包含用户的ID、用户名和密码,消息表包含消息的ID、发送者ID、接收者ID、消息内容和时间戳。
可以使用该类创建一个数据库连接,创建表,添加用户和消息,以及关闭连接。例如:
```java
ChatSystemDatabase db = new ChatSystemDatabase("jdbc:mysql://localhost:3306/chat_system", "root", "password");
db.createTables();
db.addUser(1, "alice", "password");
db.addUser(2, "bob", "password");
db.addMessage(1, 1, 2, "Hello Bob!", new Timestamp(System.currentTimeMillis()));
db.closeConnection();
```
这将创建一个名为`chat_system`的MySQL数据库,包含用户表和消息表。然后,它将添加两个用户“Alice”和“Bob”,以及一条消息从Alice发送给Bob。最后,它将关闭数据库连接。
请注意,这只是一个简单的示例,并且可能需要根据实际应用程序的要求进行修改。
相关推荐
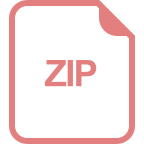
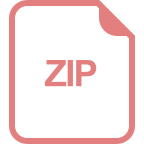
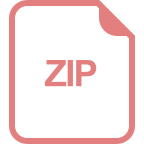















