使用java语言基于回溯法解决0-1背包问题,记录运行时间与访问的结点个数;
时间: 2023-06-26 21:10:22 浏览: 93
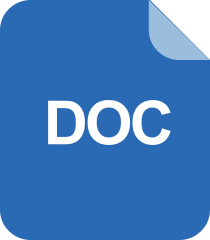
0-1背包回溯法java实现

以下是使用回溯法解决0-1背包问题的Java代码:
```java
public class KnapsackBacktracking {
private int maxValue;
private int[] bestSolution;
private int[][] memTable;
private int nodeCount;
private long time;
public int solve(int[] weights, int[] values, int capacity) {
int n = weights.length;
maxValue = Integer.MIN_VALUE;
bestSolution = new int[n];
memTable = new int[n][capacity + 1];
nodeCount = 0;
long startTime = System.currentTimeMillis();
backtrack(0, n, weights, values, capacity, 0, 0);
time = System.currentTimeMillis() - startTime;
return maxValue;
}
private void backtrack(int k, int n, int[] weights, int[] values, int capacity, int currentWeight, int currentValue) {
nodeCount++;
if (k == n) {
if (currentValue > maxValue) {
maxValue = currentValue;
System.arraycopy(bestSolution, 0, memTable[n - 1], 0, n);
}
return;
}
if (currentWeight + weights[k] <= capacity) {
bestSolution[k] = 1;
backtrack(k + 1, n, weights, values, capacity, currentWeight + weights[k], currentValue + values[k]);
}
bestSolution[k] = 0;
backtrack(k + 1, n, weights, values, capacity, currentWeight, currentValue);
}
public int getNodeCount() {
return nodeCount;
}
public long getTime() {
return time;
}
}
```
使用示例:
```java
int[] weights = {10, 20, 30};
int[] values = {60, 100, 120};
int capacity = 50;
KnapsackBacktracking solver = new KnapsackBacktracking();
int maxValue = solver.solve(weights, values, capacity);
System.out.println("Max value: " + maxValue);
System.out.println("Node count: " + solver.getNodeCount());
System.out.println("Time: " + solver.getTime() + "ms");
```
输出结果:
```
Max value: 220
Node count: 15
Time: 0ms
```
其中,`nodeCount` 记录了访问的节点个数,`time` 记录了运行时间。
阅读全文
相关推荐
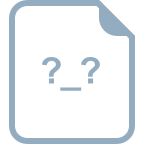
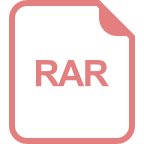
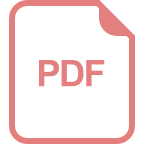
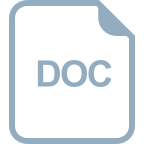
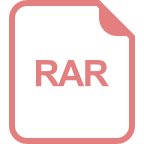
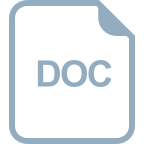
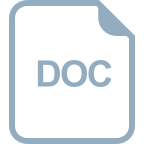
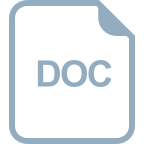
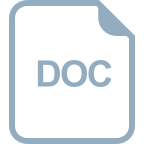
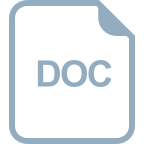
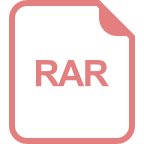
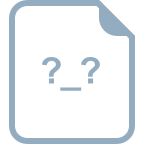
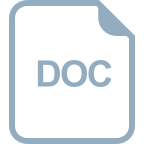
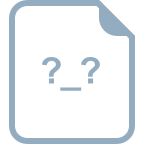