C#如何调用C++dll 函数入参 std::string 类型的方法
时间: 2024-01-21 12:04:21 浏览: 349
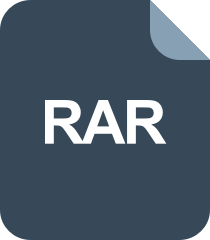
C#调用C++dll

在 C# 中调用 C++ DLL 中的函数入参为 `std::string` 类型的方法,需要使用 `MarshalAs` 特性将 C# 字符串转换为 C++ 字符串,并将其传递给 DLL 导出函数。以下是一个示例:
C++ DLL 中的函数:
```cpp
#include <string>
extern "C" {
__declspec(dllexport) void MyDllFunction(const std::string& str) {
std::string msg = "Hello " + str + " from C++!";
printf("%s\n", msg.c_str());
}
}
```
C# 代码:
```csharp
using System;
using System.Runtime.InteropServices;
class Program {
[DllImport("MyCppDll.dll")]
public static extern void MyDllFunction([MarshalAs(UnmanagedType.LPStr)] string str);
static void Main() {
string str = "World";
MyDllFunction(str);
}
}
```
在上述代码中,我们首先声明了从 C++ DLL 中导入的函数 `MyDllFunction`,它接收一个 `std::string` 类型的参数。在 C# 中,我们需要使用 `MarshalAs` 特性将 C# 字符串转换为 C++ 字符串,并将其传递给 DLL 导出函数。
在调用 `MyDllFunction` 时,我们直接传递一个 C# 字符串给它,因为 C# 字符串和 C++ `std::string` 类型可以自动转换。在 C# 中,字符串默认使用 Unicode 编码,而在 C++ 中,`std::string` 默认使用 ASCII 编码。因此,在使用 `MarshalAs` 特性时,我们需要指定字符串的编码格式为 `UnmanagedType.LPStr`,以确保 C# 字符串能够正确地转换为 C++ 字符串。
需要注意的是,如果 C++ DLL 中的函数接收的是一个指向 `std::string` 对象的指针或引用,而不是直接接收 `std::string` 对象,则需要使用 `IntPtr` 类型来传递指针或引用。在这种情况下,我们需要使用 `Marshal.StringToHGlobalAnsi` 方法将 C# 字符串转换为指向 ANSI 字符串的指针,并在调用完 DLL 导出函数后使用 `Marshal.FreeHGlobal` 方法释放内存。
阅读全文
相关推荐
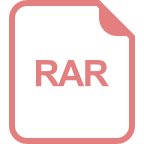
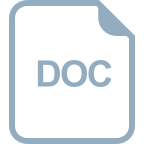







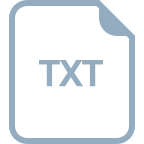
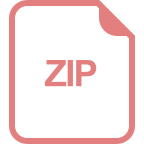


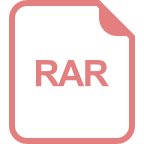
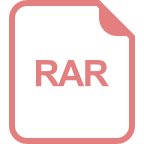
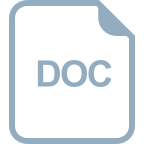
