vue计算器代码
时间: 2023-06-21 21:15:37 浏览: 137
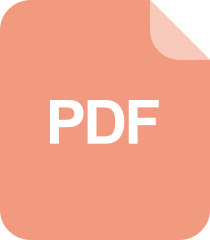
vue实现计算器功能

以下是一个简单的 Vue 计算器代码示例,包含加、减、乘、除四种基本运算:
HTML:
```
<div id="app">
<div class="calculator">
<div class="display">{{ display }}</div>
<div class="buttons">
<button v-for="button in buttons" :key="button.value" @click="handleClick(button)">{{ button.label }}</button>
</div>
</div>
</div>
```
CSS:
```
.calculator {
border: 1px solid #ccc;
width: 220px;
margin: 0 auto;
border-radius: 5px;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.2);
}
.display {
background-color: #fff;
font-size: 24px;
padding: 10px;
text-align: right;
}
.buttons {
display: flex;
flex-wrap: wrap;
}
button {
border: none;
background-color: #f5f5f5;
color: #333;
font-size: 18px;
padding: 10px;
width: 50%;
margin: -1px 0 0 -1px;
cursor: pointer;
}
button:hover {
background-color: #e6e6e6;
}
button:active {
background-color: #d9d9d9;
}
```
JavaScript:
```
new Vue({
el: '#app',
data: {
display: '0',
buttons: [
{ label: 'AC', value: 'clear' },
{ label: '+/-', value: 'negate' },
{ label: '%', value: 'percent' },
{ label: '/', value: 'divide' },
{ label: '7', value: '7' },
{ label: '8', value: '8' },
{ label: '9', value: '9' },
{ label: '*', value: 'multiply' },
{ label: '4', value: '4' },
{ label: '5', value: '5' },
{ label: '6', value: '6' },
{ label: '-', value: 'subtract' },
{ label: '1', value: '1' },
{ label: '2', value: '2' },
{ label: '3', value: '3' },
{ label: '+', value: 'add' },
{ label: '0', value: '0' },
{ label: '.', value: 'decimal' },
{ label: '=', value: 'equals' },
],
currentInput: null,
previousInput: null,
operator: null,
result: null,
decimalEntered: false,
},
methods: {
handleClick(button) {
switch (button.value) {
case 'clear':
this.clear();
break;
case 'negate':
this.negate();
break;
case 'percent':
this.percent();
break;
case 'add':
case 'subtract':
case 'multiply':
case 'divide':
this.setOperator(button.value);
break;
case 'equals':
this.calculate();
break;
case 'decimal':
this.addDecimal();
break;
default:
this.addDigit(button.value);
}
},
clear() {
this.display = '0';
this.currentInput = null;
this.previousInput = null;
this.operator = null;
this.result = null;
this.decimalEntered = false;
},
negate() {
if (this.currentInput === null) {
return;
}
if (this.currentInput === '0') {
return;
}
if (this.currentInput.startsWith('-')) {
this.currentInput = this.currentInput.substr(1);
} else {
this.currentInput = '-' + this.currentInput;
}
this.display = this.currentInput;
},
percent() {
if (this.currentInput === null) {
return;
}
this.currentInput = String(Number(this.currentInput) / 100);
this.display = this.currentInput;
},
setOperator(operator) {
if (this.currentInput === null) {
return;
}
if (this.result !== null) {
this.previousInput = this.result;
this.result = null;
} else {
this.previousInput = this.currentInput;
}
this.currentInput = null;
this.operator = operator;
this.decimalEntered = false;
},
calculate() {
if (this.currentInput === null || this.previousInput === null || this.operator === null) {
return;
}
let result;
const previous = Number(this.previousInput);
const current = Number(this.currentInput);
switch (this.operator) {
case 'add':
result = previous + current;
break;
case 'subtract':
result = previous - current;
break;
case 'multiply':
result = previous * current;
break;
case 'divide':
result = previous / current;
break;
}
this.result = result;
this.display = String(result);
this.previousInput = null;
this.currentInput = null;
this.operator = null;
this.decimalEntered = false;
},
addDigit(digit) {
if (this.currentInput === null) {
this.currentInput = digit;
} else if (this.currentInput === '0') {
this.currentInput = digit;
} else {
this.currentInput += digit;
}
this.display = this.currentInput;
},
addDecimal() {
if (this.decimalEntered) {
return;
}
if (this.currentInput === null) {
this.currentInput = '0.';
} else {
this.currentInput += '.';
}
this.display = this.currentInput;
this.decimalEntered = true;
}
}
});
```
这个计算器支持 AC(清除)、+/-(取反)、%(百分号)、加、减、乘、除四个基本运算以及小数点、数字等操作。
阅读全文
相关推荐




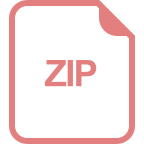
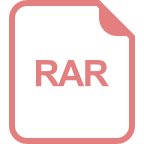
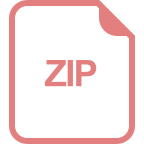
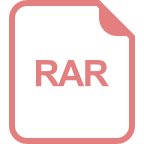
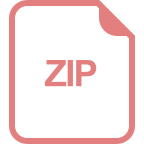
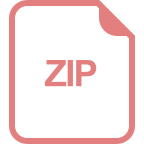
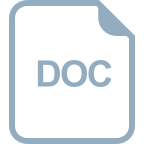



