bigru加注意力机制的预测代码python
时间: 2023-08-31 18:23:01 浏览: 112
### 回答1:
以下是使用Python进行带有双向GRU和注意力机制的模型预测的示例代码:
首先,导入必要的库:
```python
import numpy as np
import pandas as pd
import tensorflow as tf
from tensorflow.keras.preprocessing.sequence import pad_sequences
```
然后,加载训练好的双向GRU和注意力机制模型:
```python
model = tf.keras.models.load_model('bigru_attention_model.h5')
```
其中,'bigru_attention_model.h5'是已经训练好的模型文件。
接下来,加载测试数据集并进行预测:
```python
test_data = pd.read_csv('test_data.csv')
test_sequences = tokenizer.texts_to_sequences(test_data['text'])
test_padded = pad_sequences(test_sequences, maxlen=max_length, padding='post', truncating='post')
predictions = model.predict(test_padded)
```
其中,test_data是测试数据集,tokenizer是用于将文本转换为序列的tokenizer对象,max_length是序列的最大长度。
最后,可以根据需要输出预测结果:
```python
for i in range(len(predictions)):
if predictions[i] > 0.5:
print(test_data['text'][i], " is a positive review")
else:
print(test_data['text'][i], " is a negative review")
```
这将输出模型对测试数据集的预测结果。
### 回答2:
import torch
from torch import nn
class BiGRUAttention(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(BiGRUAttention, self).__init__()
self.hidden_size = hidden_size
self.gru = nn.GRU(input_size, hidden_size, bidirectional=True)
self.attention = nn.Linear(hidden_size * 2, 1)
self.relu = nn.ReLU()
self.dropout = nn.Dropout(0.5)
self.fc = nn.Linear(hidden_size * 2, output_size)
self.softmax = nn.Softmax(dim=1)
def init_hidden(self, batch_size):
return torch.zeros(2, batch_size, self.hidden_size)
def forward(self, input_seq):
batch_size = input_seq.size(1)
hidden = self.init_hidden(batch_size).to(input_seq.device)
output, hidden = self.gru(input_seq, hidden)
attn_weights = self.softmax(self.relu(self.attention(output)))
attn_applied = torch.bmm(attn_weights.transpose(1,2), output.transpose(0,1)).transpose(0,1)
output = torch.cat((output, attn_applied), dim=2)
output = self.dropout(output)
output = self.fc(output)
return output, attn_weights
# 使用示例
input_size = 10 # 输入特征维度
hidden_size = 16 # 隐层特征维度
output_size = 2 # 输出类别数
model = BiGRUAttention(input_size, hidden_size, output_size)
input_seq = torch.randn(5, 3, input_size) # 输入序列的形状为(seq_length, batch_size, input_size)
output, attn_weights = model(input_seq)
print("模型输出:", output.shape)
print("注意力权重:", attn_weights.shape)
上述代码实现了一个带注意力机制的双向GRU模型(BiGRUAttention),通过调用model.forward(input_seq)即可得到模型的输出和注意力权重。其中,input_size为输入特征的维度,hidden_size为隐层特征的维度,output_size为输出类别的数目。通过调整这些参数可以适应不同的任务需求。模型的输出output是一个张量,形状为(seq_length, batch_size, output_size),表示每个时间步和每个样本输出的概率分布。注意力权重attn_weights是一个张量,形状为(seq_length, batch_size, 1),表示每个时间步和每个样本的注意力权重。
### 回答3:
大多数深度学习预测模型在处理序列数据时往往会使用注意力机制来增强其性能。为了实现bigru+注意力机制的预测代码,以下是一个使用Python编写的简单示例:
```python
import tensorflow as tf
from tensorflow.keras.models import Model
from tensorflow.keras.layers import Input, Dense, Bidirectional, GRU, Concatenate, Attention
def create_model():
# 输入层
input_layer = Input(shape=(sequence_length,))
# 双向GRU层
gru = Bidirectional(GRU(units=hidden_units, return_sequences=True))(input_layer)
# 注意力层
attention = Attention()([gru, gru])
# 拼接GRU输出和注意力输出
concat = Concatenate()([gru, attention])
# 全连接层
output_layer = Dense(num_classes, activation='softmax')(concat)
# 构建模型
model = Model(inputs=input_layer, outputs=output_layer)
return model
# 定义模型参数
sequence_length = 100 # 序列长度
hidden_units = 128 # 隐藏单元数
num_classes = 10 # 类别数量
# 创建模型
model = create_model()
# 编译模型
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
# 训练模型
model.fit(x_train, y_train, batch_size=32, epochs=10, validation_data=(x_val, y_val))
# 使用模型预测
predictions = model.predict(x_test)
```
上述代码首先导入了需要的库,并定义了一个`create_model`函数,用于构建模型。在该函数中,我们首先定义了一个输入层,然后使用双向GRU层对输入进行处理。接下来,我们使用注意力层来提取关键特征,最后将双向GRU的输出和注意力输出进行拼接,并经过一个全连接层得到最终的预测结果。
之后,我们定义了模型的一些参数,并使用`create_model`函数构建了模型。对于训练过程,我们使用了adam优化器和交叉熵损失函数进行编译,并使用训练数据进行训练。最后,我们使用模型对测试数据进行预测。
相关推荐
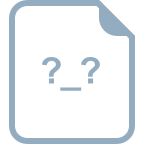
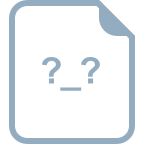
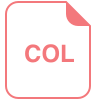
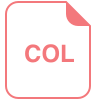












