生成一段有关加密技术的C++代码
时间: 2023-03-11 20:13:35 浏览: 155
这是一段使用加密技术的C代码:#include <stdio.h>
#include <stdlib.h>
#include <string.h>int main()
{
char plaintext[100] = "Hello, World!"; //明文
char ciphertext[100]; //密文
int key = 10; //加密钥匙
// 加密算法
int i;
for(i = 0; i < strlen(plaintext); i++){
ciphertext[i] = plaintext[i] ^ key;
} printf("plaintext: %s\n", plaintext);
printf("ciphertext: %s\n", ciphertext); return 0;
}
相关问题
RSA公钥加密算法c++实现
RSA (Rivest-Shamir-Adleman) 公钥加密算法是一种非对称加密技术,它基于大数分解的难度,安全性非常高。在C++中实现RSA的关键步骤包括生成密钥对、加密和解密过程。以下是基本的步骤:
1. 密钥生成:
- 选择两个大素数p和q,并计算它们的乘积n = p * q。
- 计算欧拉函数φ(n) = (p-1) * (q-1),然后选择一个小于φ(n)的整数e,满足gcd(e, φ(n)) = 1作为公钥指数。
- 求出e关于φ(n)的模逆数d,即满足 d * e ≡ 1 mod φ(n),d就是私钥。
2. 加密(公钥操作):
- 对明文消息m取模n,得到整数0 <= m < n。
- 使用公式 c ≡ m^e mod n 来计算密文c。
3. 解密(私钥操作):
- 将密文c使用私钥d进行解密,计算 m ≡ c^d mod n。
4. 实现时会涉及大数运算库(如GMP),因为直接操作大整数可能会导致溢出,所以需要高效的算法和数据结构处理。
注意:实际应用中,为了保护私钥的安全性,通常不会将其公开,而是存储在安全的地方,比如文件系统的一个特定位置,或者内存中由受信任的代码段管理。
用c/c++写一段古典密码算法的实现代码
在C++中,我们可以实现简单的Caesar Cipher(凯撒密码)算法,这是一个最基础的替换式加密技术。这种算法通过将明文中的每个字符按照固定的位移进行替换,生成密文。下面是一个简单的凯撒密码实现:
```cpp
#include <iostream>
using namespace std;
char shift(char c, int s) {
if (c >= 'A' && c <= 'Z') // 大写字母处理
return static_cast<char>((c - 'A' + s) % 26 + 'A');
else if (c >= 'a' && c <= 'z') // 小写字母处理
return static_cast<char>((c - 'a' + s) % 26 + 'a');
else
return c; // 对其他字符不做改动
}
void caesar_cipher(string plaintext, int shift_amount) {
string ciphertext = "";
for (char c : plaintext) {
ciphertext += shift(c, shift_amount);
}
cout << "Ciphertext: " << ciphertext << endl;
}
int main() {
string input;
cout << "Enter the plaintext: ";
getline(cin, input);
int key;
cout << "Enter the shift amount (1-25): ";
cin >> key;
caesar_cipher(input, key);
return 0;
}
```
在这个代码中,`shift`函数负责单个字符的加密,`caesar_cipher`函数则遍历整个字符串并应用该函数。用户需要输入明文和位移量(通常范围为1到25),然后会得到相应的密文。
阅读全文
相关推荐
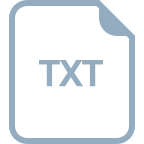
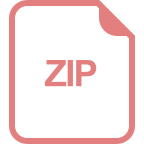
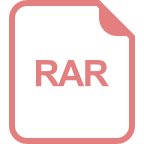
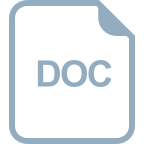
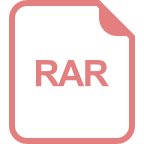
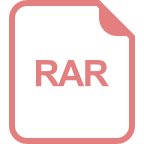
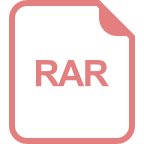
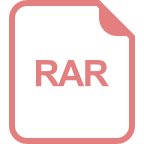
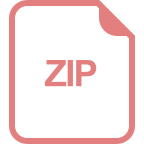
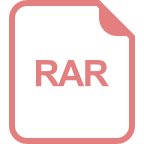
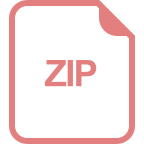
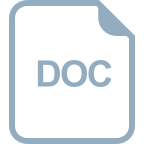
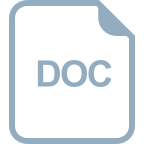
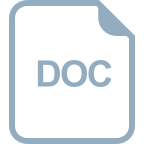