请详细介绍单例模式,单例模式的作用,使用场景,以及单例模式的分类,最后面针对不同分类请写出对应的代码
时间: 2023-06-04 08:07:49 浏览: 99
单例模式是一种创建型设计模式,主要作用是确保某个类只有一个实例,同时提供对该实例的全局访问。
单例模式的使用场景很多,比如需要全局控制某个资源、需要频繁创建和销毁的对象、需要使用应用程序配置信息的对象等等。
单例模式可以分为以下几种:
1. 懒汉式单例模式:在第一次使用时才创建单例对象。
2. 饿汉式单例模式:在类加载的时候就创建单例对象。
3. 双重检查锁定单例模式:结合了懒汉式和饿汉式的优点,既能在需要时创建单例对象,同时又能保证线程安全。
4. 登记式单例模式:把所有的单例对象都放在一个注册表中,保证在任何地方都可以随时访问。
下面是几种不同分类对应的代码实现:
1. 懒汉式单例模式:
```java
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static synchronized Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
```
2. 饿汉式单例模式:
```java
public class Singleton {
private static Singleton instance = new Singleton();
private Singleton() {}
public static Singleton getInstance() {
return instance;
}
}
```
3. 双重检查锁定单例模式:
```java
public class Singleton {
private volatile static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
synchronized (Singleton.class) {
if (instance == null) {
instance = new Singleton();
}
}
}
return instance;
}
}
```
4. 登记式单例模式:
```java
public class Singleton {
private static Map<String, Singleton> map = new HashMap<>();
static {
Singleton instance = new Singleton();
map.put(instance.getClass().getName(), instance);
}
protected Singleton() {}
public static Singleton getInstance(String name) {
if (name == null) {
name = Singleton.class.getName();
}
if (map.get(name) == null) {
try {
map.put(name, (Singleton) Class.forName(name).newInstance());
} catch (InstantiationException | IllegalAccessException | ClassNotFoundException e) {
e.printStackTrace();
}
}
return map.get(name);
}
}
```
阅读全文
相关推荐
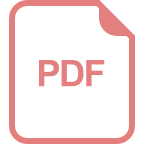
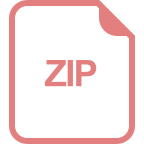
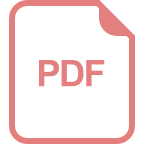















