vue2 二叉树页面d3.js
时间: 2023-09-15 08:20:04 浏览: 102
可以使用 d3.js 来实现 vue2 二叉树页面。
首先,你需要在 Vue 项目中安装 d3.js:
```
npm install d3
```
然后,在 Vue 组件中引入 d3.js:
```
import * as d3 from 'd3';
```
接下来,你可以使用 d3.js 创建 SVG 元素并在其中绘制二叉树。例如,下面是一个简单的实现:
```
<template>
<div ref="svgContainer"></div>
</template>
<script>
export default {
data() {
return {
treeData: {
name: 'A',
children: [
{
name: 'B',
children: [
{
name: 'D'
},
{
name: 'E'
}
]
},
{
name: 'C',
children: [
{
name: 'F'
},
{
name: 'G'
}
]
}
]
}
};
},
mounted() {
const svg = d3.select(this.$refs.svgContainer)
.append('svg')
.attr('width', '100%')
.attr('height', '100%')
.attr('viewBox', `0 0 ${this.$refs.svgContainer.offsetWidth} ${this.$refs.svgContainer.offsetHeight}`);
const treeLayout = d3.tree()
.size([this.$refs.svgContainer.offsetHeight, this.$refs.svgContainer.offsetWidth - 100]);
const root = d3.hierarchy(this.treeData);
const links = treeLayout(root).links();
const nodes = treeLayout(root).descendants();
svg.selectAll('.link')
.data(links)
.enter()
.append('path')
.attr('class', 'link')
.attr('d', d => `M${d.source.y},${d.source.x}L${d.target.y},${d.target.x}`);
const node = svg.selectAll('.node')
.data(nodes)
.enter()
.append('g')
.attr('class', 'node')
.attr('transform', d => `translate(${d.y},${d.x})`);
node.append('circle')
.attr('r', 10);
node.append('text')
.attr('x', -10)
.attr('y', 5)
.text(d => d.data.name);
}
};
</script>
<style scoped>
.node {
font: 12px sans-serif;
}
.link {
fill: none;
stroke: #ccc;
stroke-width: 1.5px;
}
</style>
```
在这个例子中,我们使用了 `d3.tree()` 函数来创建一个树形布局,并使用 `size()` 方法指定了布局的大小。我们还使用了 `d3.hierarchy()` 函数来将数据转换为层次结构。然后,我们使用 `treeLayout()` 方法将层次结构传递给树形布局并获取节点和链接。最后,我们使用 D3.js 的数据绑定来绘制节点和链接。
这只是一个简单的例子,你可以根据自己的需求来修改和扩展它。
阅读全文
相关推荐
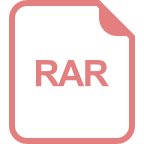
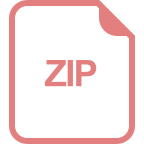
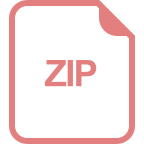
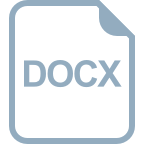
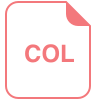
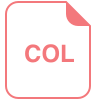

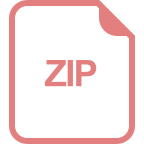
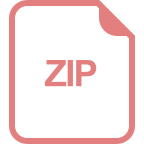