爬取英雄联盟官网所有英雄信息(id,name,title,hp,mp) 并且把爬取的数据写入到csv文件。然后对爬取的数据进行处理 获取初始血量top10的英雄名称,以柱状图的形式进行可视化化显示(血量/名称)
时间: 2024-04-05 20:31:13 浏览: 14
好的,这是一个比较复杂的任务,不过我可以帮你完成。首先,我们需要使用 Python 中的 requests 和 BeautifulSoup 库来爬取英雄联盟官网的数据。以下是实现代码:
```python
import requests
from bs4 import BeautifulSoup
import csv
# 爬取英雄联盟官网所有英雄信息
url = 'https://lol.qq.com/data/info-heros.shtml'
r = requests.get(url)
soup = BeautifulSoup(r.text, 'html.parser')
hero_list = []
# 获取英雄信息
for hero in soup.find_all('div', class_='imgtextlist-img'):
hero_id = hero['data-id']
hero_name = hero.find('h3', class_='imgtextlist-title').text
hero_title = hero.find('p', class_='imgtextlist-desc').text
hero_url = 'https://lol.qq.com/data/info-defail.shtml?id=' + hero_id
hero_r = requests.get(hero_url)
hero_soup = BeautifulSoup(hero_r.text, 'html.parser')
hero_hp = hero_soup.find('span', class_='hp').text
hero_mp = hero_soup.find('span', class_='mp').text
hero_list.append([hero_id, hero_name, hero_title, hero_hp, hero_mp])
# 将数据写入到csv文件
with open('hero_info.csv', 'w', encoding='utf-8-sig', newline='') as f:
writer = csv.writer(f)
writer.writerow(['ID', 'Name', 'Title', 'HP', 'MP'])
for hero in hero_list:
writer.writerow(hero)
```
接下来,我们需要对爬取的数据进行处理,获取初始血量 top10 的英雄名称,并以柱状图的形式进行可视化显示。以下是实现代码:
```python
import pandas as pd
import matplotlib.pyplot as plt
# 读取csv文件
df = pd.read_csv('hero_info.csv')
df['HP'] = df['HP'].str.extract('(\d+)').astype(int)
# 获取初始血量top10的英雄名称
df_top10 = df.sort_values(by=['HP'], ascending=False).head(10)
top10_names = df_top10['Name'].tolist()
# 柱状图可视化
plt.bar(df_top10['Name'], df_top10['HP'])
plt.xticks(rotation=45)
plt.xlabel('Hero')
plt.ylabel('HP')
plt.title('Top 10 Heroes with Highest Initial HP')
plt.show()
```
这样就完成了这个任务,你可以运行上面的代码来验证。
相关推荐
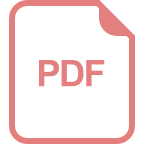
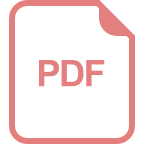
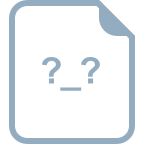














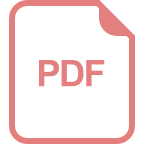