在IDEA用Java设计一个简单的学生选课系统,并可以完成添加、删除、修改、查询相关信息 。 说明: 可以设计以下类: 描述课程基本信息类(课程编号,课程名,学分 ); 描述学生基本信息类(学号,姓名,专业方向 ); 记录选课结果的类(课程,选课的学生,课程最多人数,当前人数 ); 测试类(设定或输入最多人数,创建课程对象,创建选课学生对象,创建选课对象,显示选课情况 )
时间: 2024-03-04 13:51:37 浏览: 78
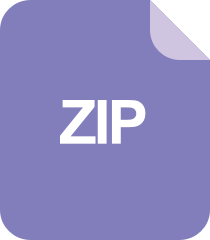
本人菜鸟一个,类似于课程设计的Javaee项目,基于SSH的简易选课系统,有兴趣的可以拿去扩展或者修改.zip
好的,这是一个Java的面向对象设计问题,我会尽力回答。
首先,我们需要设计三个类,分别是Course、Student和Selection。
Course类用于描述课程的基本信息,包括课程编号、课程名和学分。代码如下:
```java
public class Course {
private String id; // 课程编号
private String name; // 课程名
private int credit; // 学分
public Course(String id, String name, int credit) {
this.id = id;
this.name = name;
this.credit = credit;
}
// getter and setter methods
}
```
Student类用于描述学生的基本信息,包括学号、姓名和专业方向。代码如下:
```java
public class Student {
private String id; // 学号
private String name; // 姓名
private String major; // 专业方向
public Student(String id, String name, String major) {
this.id = id;
this.name = name;
this.major = major;
}
// getter and setter methods
}
```
Selection类用于记录选课结果,包括选课的课程、学生、课程最多人数和当前人数。代码如下:
```java
public class Selection {
private Course course; // 选课的课程
private Student student; // 选课的学生
private int maxNum; // 课程最多人数
private int currentNum; // 当前人数
public Selection(Course course, Student student, int maxNum, int currentNum) {
this.course = course;
this.student = student;
this.maxNum = maxNum;
this.currentNum = currentNum;
}
// getter and setter methods
}
```
最后,我们需要一个测试类,用于测试我们的选课系统。测试类中需要设定或输入最多人数,创建课程对象、创建学生对象、创建选课对象,并显示选课情况。代码如下:
```java
import java.util.ArrayList;
import java.util.List;
public class Test {
public static void main(String[] args) {
// 设定最大选课人数
int maxNum = 30;
// 创建课程对象
Course javaCourse = new Course("001", "Java程序设计", 3);
Course databaseCourse = new Course("002", "数据库原理", 4);
// 创建学生对象
Student student1 = new Student("1001", "张三", "计算机科学与技术");
Student student2 = new Student("1002", "李四", "软件工程");
Student student3 = new Student("1003", "王五", "信息安全");
// 创建选课对象
List<Selection> selections = new ArrayList<>();
selections.add(new Selection(javaCourse, student1, maxNum, 1));
selections.add(new Selection(javaCourse, student2, maxNum, 2));
selections.add(new Selection(databaseCourse, student2, maxNum, 1));
selections.add(new Selection(databaseCourse, student3, maxNum, 2));
// 显示选课情况
for (Selection selection : selections) {
System.out.println("课程:" + selection.getCourse().getName());
System.out.println("学生:" + selection.getStudent().getName());
System.out.println("当前人数:" + selection.getCurrentNum() + "/" + selection.getMaxNum());
System.out.println();
}
}
}
```
以上就是一个简单的学生选课系统的设计和实现。当然,还有很多方面需要完善,比如增加选课、退课功能,增加管理员账号等等。
阅读全文
相关推荐







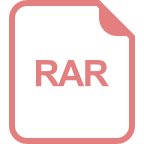
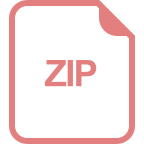
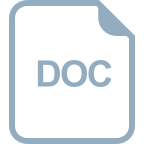
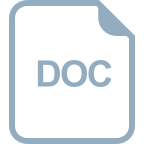
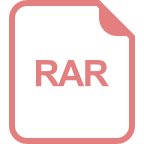
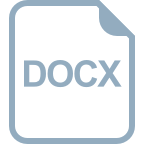
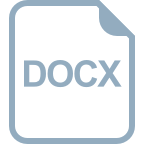
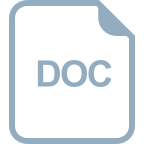
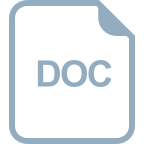