请用R语言对泰坦尼克号数据集进行数据探索,数据清洗与预处理,特征工程,数据建模与评估,模型融合与评估,数据预测。
时间: 2024-03-23 15:40:25 浏览: 88
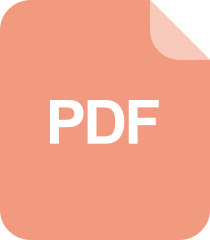
数据分析 R 语言
好的,我将为您提供一个大致的R语言代码流程,供您参考。
1. 数据探索与清洗
```R
# 导入数据
titanic <- read.csv("train.csv")
# 查看数据基本信息
str(titanic)
# 查看数据摘要信息
summary(titanic)
# 查看数据前几行
head(titanic)
# 查看数据后几行
tail(titanic)
# 查看每列的缺失值情况
sapply(titanic, function(x) sum(is.na(x)))
# 删除无用的列
titanic$PassengerId <- NULL
titanic$Name <- NULL
titanic$Ticket <- NULL
titanic$Cabin <- NULL
```
2. 特征工程
```R
# 对数据进行特征工程
# 将性别转化为二元变量
titanic$Sex <- as.integer(titanic$Sex == "female")
# 将登船港口转化为三元变量
titanic$Embarked[titanic$Embarked == "S"] <- 1
titanic$Embarked[titanic$Embarked == "C"] <- 2
titanic$Embarked[titanic$Embarked == "Q"] <- 3
titanic$Embarked[is.na(titanic$Embarked)] <- 0
# 将年龄缺失值填充为中位数
titanic$Age[is.na(titanic$Age)] <- median(titanic$Age, na.rm = TRUE)
# 将船票价格分为四个等级
titanic$FareLevel <- cut(titanic$Fare, breaks = c(0, 10, 50, 100, Inf), labels = c(1, 2, 3, 4))
# 删除处理后的列
titanic$Fare <- NULL
```
3. 数据建模与评估
```R
# 划分训练集和测试集
set.seed(123)
train_index <- sample(1:nrow(titanic), 0.7 * nrow(titanic))
train_data <- titanic[train_index, ]
test_data <- titanic[-train_index, ]
# 使用随机森林进行建模和预测
library(randomForest)
model <- randomForest(as.factor(Survived) ~ ., data = train_data)
pred <- predict(model, newdata = test_data)
# 计算模型评估指标
library(caret)
confusionMatrix(pred, as.factor(test_data$Survived))
```
4. 模型融合与评估
```R
# 使用GBM进行模型融合
library(gbm)
gbm_model <- gbm(as.factor(Survived) ~ ., data = train_data, n.trees = 500, interaction.depth = 3, shrinkage = 0.01, verbose = FALSE)
# 使用融合模型进行预测
gbm_pred <- predict.gbm(gbm_model, newdata = test_data, n.trees = 500)
# 对两个模型进行加权融合
final_pred <- (pred + gbm_pred) / 2
# 计算融合模型的评估指标
confusionMatrix(as.factor(ifelse(final_pred > 0.5, 1, 0)), as.factor(test_data$Survived))
```
5. 数据预测
```R
# 对测试集进行预测,并保存结果为csv文件
test_data$FareLevel <- cut(test_data$Fare, breaks = c(0, 10, 50, 100, Inf), labels = c(1, 2, 3, 4))
test_data$Fare <- NULL
test_data$Sex <- as.integer(test_data$Sex == "female")
test_data$Embarked[test_data$Embarked == "S"] <- 1
test_data$Embarked[test_data$Embarked == "C"] <- 2
test_data$Embarked[test_data$Embarked == "Q"] <- 3
test_data$Embarked[is.na(test_data$Embarked)] <- 0
test_pred <- predict(model, newdata = test_data)
test_output <- data.frame(PassengerId = (892:1309), Survived = test_pred)
write.csv(test_output, file = "titanic_pred.csv", row.names = FALSE)
```
以上就是一个大致的R语言代码流程,具体实现可能需要根据数据的具体情况进行调整和修改。
阅读全文
相关推荐
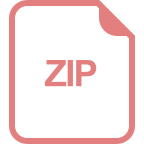
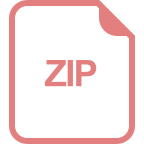
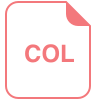
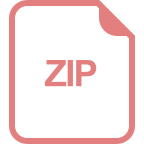
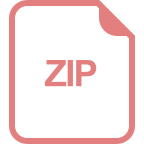
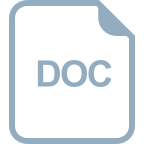
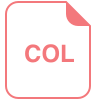
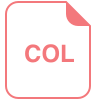
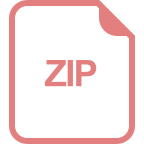
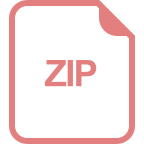
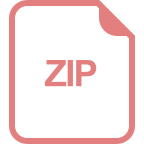
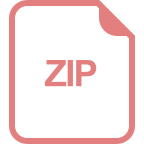
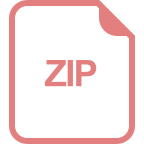
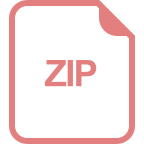