:使用Pipe创建管道,创建一个子进程,子进程向父进程发送消息“I am your son!”,父进程接收到子进程的消息后,显示在屏幕上,并向子进程发送“I am your father!”。子进程接收到父进程的消息并显示在屏幕上。 任务2:使用系统调用 pipe ( ) 建立一条管道线;两个子进程 P1 和 P2 分别向管道各写一句话 Child 1 is sending a message! Child 2 is sending a message! 而父进程则从管道中读出来自于两个子进程的信息,显示在屏幕上。 任务3:能否创建一条管道,实现两个子进程之间的通信,如果可以,请实现并给出代码。如果不可以,请说明理由
时间: 2023-06-05 10:06:07 浏览: 374
回答:非常感谢您的提问!首先回答第一个问题:根据您的要求,我可以帮助您完成使用Pipe创建管道的进程通信任务。以下是示范代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
int main(){
int pipefd[2];
pid_t pid;
char buf[20];
if(pipe(pipefd)<0){
perror("pipe error");
exit(EXIT_FAILURE);
}
pid=fork();
if(pid<0){
perror("fork error");
exit(EXIT_FAILURE);
}
if(pid==0){
close(pipefd[0]);
write(pipefd[1],"I am your son!",14);
close(pipefd[1]);
exit(EXIT_SUCCESS);
}
else{
close(pipefd[1]);
read(pipefd[0],buf,20);
printf("%s\n",buf);
write(pipefd[1],"I am your father!",17);
close(pipefd[0]);
wait(NULL);
exit(EXIT_SUCCESS);
}
}
```
以上代码使用系统调用 pipe() 建立了一个管道,然后创建了一个子进程,子进程向父进程写入"I am your son!”消息,父进程接收到子进程的消息后,在屏幕上显示出来,并向子进程写入“I am your father!"的消息,最后子进程接收到父进程的消息并同样显示在屏幕上。
接下来回答第二个问题:根据您的要求,我可以帮您完成使用系统调用pipe()建立一条管道线的进程通信任务。以下是示范代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
int main(){
int pipefd[2];
pid_t pid1,pid2;
char buf1[30];
char buf2[30];
if(pipe(pipefd)<0){
perror("pipe error");
exit(EXIT_FAILURE);
}
pid1=fork();
if(pid1<0){
perror("fork1 error");
exit(EXIT_FAILURE);
}
if(pid1==0){
close(pipefd[0]);
write(pipefd[1],"Child 1 is sending a message!",30);
close(pipefd[1]);
exit(EXIT_SUCCESS);
}
pid2=fork();
if(pid2<0){
perror("fork2 error");
exit(EXIT_FAILURE);
}
if(pid2==0){
close(pipefd[0]);
write(pipefd[1],"Child 2 is sending a message!",30);
close(pipefd[1]);
exit(EXIT_SUCCESS);
}
close(pipefd[1]);
read(pipefd[0],buf1,30);
printf("%s\n",buf1);
read(pipefd[0],buf2,30);
printf("%s\n",buf2);
close(pipefd[0]);
waitpid(pid1,NULL,0);
waitpid(pid2,NULL,0);
exit(EXIT_SUCCESS);
}
```
以上代码创建了两个子进程P1和P2,它们分别向管道写入"Child 1 is sending a message!"和"Child 2 is sending a message!"的消息,父进程从管道中读出来自两个子进程的信息,并在屏幕上显示出来。
最后回答第三个问题:可以创建一条管道,实现两个子进程之间的通信。理由是,管道是一种具有两个文件描述符的文件,它允许进程之间进行单向或双向通信。利用这个特性,我们可以创建两个子进程,一个子进程从管道的一侧写入信息,另一个子进程从另一侧读取信息,从而实现进程间通信。以下是示范代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
int main(){
int pipefd[2];
pid_t pid1,pid2;
char buf[30];
if(pipe(pipefd)<0){
perror("pipe error");
exit(EXIT_FAILURE);
}
pid1=fork();
if(pid1<0){
perror("fork1 error");
exit(EXIT_FAILURE);
}
if(pid1==0){
close(pipefd[0]);
write(pipefd[1],"Hello, I'm Child 1!",19);
close(pipefd[1]);
exit(EXIT_SUCCESS);
}
pid2=fork();
if(pid2<0){
perror("fork2 error");
exit(EXIT_FAILURE);
}
if(pid2==0){
close(pipefd[1]);
read(pipefd[0],buf,30);
printf("%s\n",buf);
close(pipefd[0]);
exit(EXIT_SUCCESS);
}
close(pipefd[0]);
close(pipefd[1]);
waitpid(pid1,NULL,0);
waitpid(pid2,NULL,0);
exit(EXIT_SUCCESS);
}
```
以上代码创建了两个子进程P1和P2,P1向管道写入信息“Hello, I'm Child 1!",P2从管道中读出信息并在屏幕上显示出来。这个例子展示了如何利用管道实现两个子进程之间的通信。
阅读全文
相关推荐
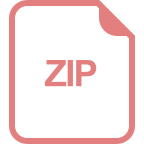
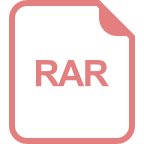
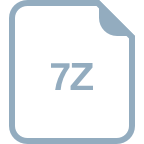

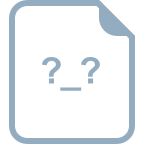
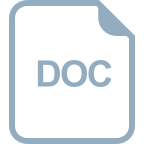
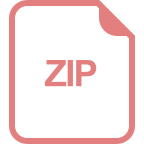
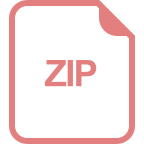
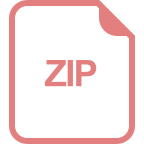
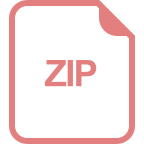
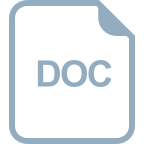
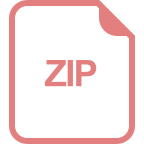
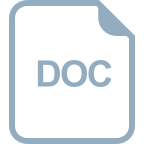
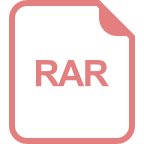
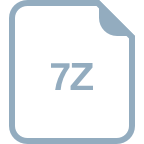
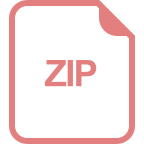
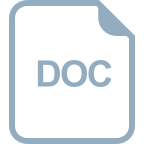