用C语言编写使用顺序队列实现上述数据进队和出队的操作,每次进队和出队操作后需要遍历队列。
时间: 2024-03-13 19:43:00 浏览: 60
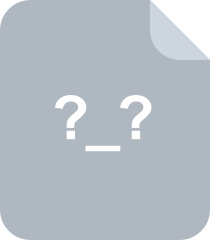
c语言实现队列,出队入队等操作
以下是使用顺序队列实现上述数据进队和出队的操作的C语言代码。每次进队和出队操作后都会遍历队列。
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 4 // 队列的最大长度
typedef struct {
int data[MAXSIZE]; // 存储队列元素
int front; // 队头指针
int rear; // 队尾指针
} Queue;
// 初始化队列
void initQueue(Queue *queue) {
queue->front = 0;
queue->rear = 0;
}
// 判断队列是否为空
int isEmpty(Queue *queue) {
return queue->front == queue->rear;
}
// 判断队列是否已满
int isFull(Queue *queue) {
return (queue->rear + 1) % MAXSIZE == queue->front;
}
// 入队
void enQueue(Queue *queue, int element) {
if (isFull(queue)) {
printf("队列已满,入队失败!\n");
return;
}
queue->data[queue->rear] = element;
queue->rear = (queue->rear + 1) % MAXSIZE;
}
// 出队
void deQueue(Queue *queue) {
if (isEmpty(queue)) {
printf("队列为空,出队失败!\n");
return;
}
printf("出队的元素是:%d\n", queue->data[queue->front]);
queue->front = (queue->front + 1) % MAXSIZE;
}
// 遍历队列
void traverseQueue(Queue *queue) {
if (isEmpty(queue)) {
printf("队列为空!\n");
return;
}
printf("队列中的元素有:");
int i = queue->front;
while (i != queue->rear) {
printf("%d ", queue->data[i]);
i = (i + 1) % MAXSIZE;
}
printf("\n");
}
int main() {
Queue queue;
initQueue(&queue);
// 进队
enQueue(&queue, 1);
enQueue(&queue, 2);
enQueue(&queue, 3);
enQueue(&queue, 4);
// 遍历队列
traverseQueue(&queue);
// 出队
deQueue(&queue);
deQueue(&queue);
// 遍历队列
traverseQueue(&queue);
return 0;
}
```
阅读全文
相关推荐
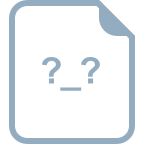

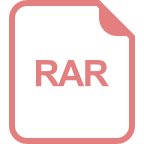
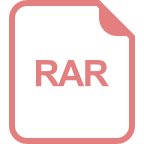
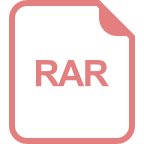
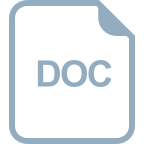
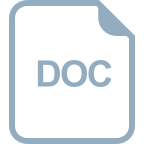
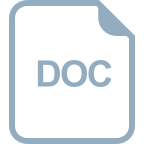
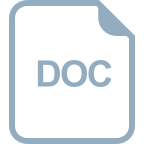
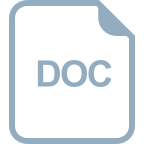






