以下代码报错,看看哪里出了问题import requests from bs4 import BeautifulSoup # 获取双色球历史数据的url url = 'https://datachart.500.com/ssq/history/newinc/history.php?start=03001&end=21115' # 发送GET请求获取网页内容 response = requests.get(url) # 使用BeautifulSoup解析网页内容 soup = BeautifulSoup(response.content, 'html.parser') # 获取开奖号码和开奖日期 trs = soup.find_all('tr')[2:] result_dict = {} for tr in trs: tds = tr.find_all('td') date = tds[0].text nums = [int(td.text) for td in tds[1:-1]] blue = int(tds[-1].text) result_dict[date] = tuple(nums + [blue]) # 对每个中奖号码进行统计 count_dict = {} for nums in result_dict.values(): for num in nums: count_dict[num] = count_dict.get(num, 0) + 1 # 输出结果 print('历史数据统计结果:') for num, count in count_dict.items(): print('{}出现了{}次'.format(num, count))
时间: 2023-03-02 14:41:29 浏览: 108
这段代码的问题在于缺少语句之间的分隔符。应该使用分号 (;) 或换行符将两个语句分开。此外,如果没有将这两个语句包含在函数或类定义中,则需要在它们之前添加模块导入语句。
正确的代码应该是这样的:
```python
import requests
from bs4 import BeautifulSoup
```
这将导入 requests 和 BeautifulSoup 模块,并使它们可在当前的 Python 脚本中使用。
相关问题
import requestsfrom bs4 import BeautifulSoup# 发送 GET 请求获取网页内容url = 'https://buff.163.com/market/goods?goods_id=35864&from=market#tab=selling'res = requests.get(url)# 使用 BeautifulSoup 解析 HTMLsoup = BeautifulSoup(res.text, 'html.parser')# 查找手套武器箱价格并打印price = soup.find('span', {'class': 'price'}).textprint('手套武器箱价格为:' + price)
这段代码的问题在于第一行 `import requestsfrom bs4 import BeautifulSoup`,`requests` 和 `bs4` 库的导入应该在两行中分开导入,即应该写成:
```python
import requests
from bs4 import BeautifulSoup
# 发送 GET 请求获取网页内容
url = 'https://buff.163.com/market/goods?goods_id=35864&from=market#tab=selling'
res = requests.get(url)
# 使用 BeautifulSoup 解析 HTML
soup = BeautifulSoup(res.text, 'html.parser')
# 查找手套武器箱价格并打印
price = soup.find('span', {'class': 'price'}).text
print('手套武器箱价格为:' + price)
```
请将代码复制粘贴到您的编辑器中并重新运行。
import requestsfrom bs4 import BeautifulSoup# 请求页面并获取页面内容url = "https://www.example.com"response = requests.get(url)html = response.text# 使用BeautifulSoup解析页面soup = BeautifulSoup(html, "html.parser")# 获取需要的信息info = soup.find("div", {"class": "info"})print(info.text)
这是一个简单的爬虫代码,它的功能是请求指定网址的页面并解析页面中的HTML代码,最后获取指定标签的文本内容并打印出来。这个代码使用了requests和BeautifulSoup库,其中requests库用于发送HTTP请求,BeautifulSoup库用于解析HTML代码。具体步骤如下:
1. 导入requests和BeautifulSoup库。
2. 指定需要爬取的网址,并使用requests库发送HTTP请求。
3. 获取响应对象中的HTML代码,并使用BeautifulSoup库解析HTML代码。
4. 使用find()函数查找指定的标签,并获取该标签的文本内容。
5. 打印获取到的文本内容。
需要注意的是,这个代码只是一个简单的示例,实际爬虫代码需要考虑到各种情况,如反爬虫措施、异常处理、数据存储等。
相关推荐
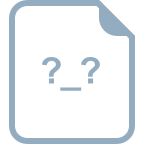
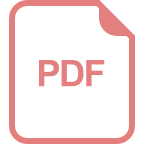
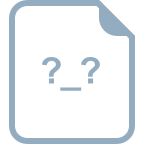













