撰写一个cache模拟器,模拟cache不同配置下的命中率。 语言不限,C/C++/Java/Python等各种语言均可。 使用提供的trace文件进行评测。 要求能输入以下Cache配置参数对不同配置下的Cache命中率进行统计。 (1) Sizes: 1024, 2048, 4096, 8192, 16384 bytes (2)Block size: 8 bytes,16 bytes,32bytes (3)Associativities: Direct Mapped, 2-way, 4-way, 8-way (4)Replacement Policy: LRU,FIFO,Random 不用做图形化输入界面,只要能输入参数即可。 附件提供的trace.txt文件为cache访问的地址序列,每行是一个16进制的访存地址(32位的字节地址)。假设cache初始化时为空,要求模拟出访问全部地址之后总的命中率是多少。
时间: 2024-04-01 12:32:16 浏览: 20
好的,我可以为您解答这个问题。首先,cache模拟器的实现需要了解一些基本的计算机组成原理和cache的工作原理。在这里我假设您已经了解了这些知识。
以下是一个Python实现的cache模拟器,可以根据输入的参数模拟不同配置下的cache命中率:
```python
import sys
# Cache configuration parameters
sizes = [1024, 2048, 4096, 8192, 16384]
block_sizes = [8, 16, 32]
associativities = [1, 2, 4, 8]
replacement_policies = ["LRU", "FIFO", "Random"]
# Function to simulate cache access
def simulate_cache(cache_size, block_size, associativity, replacement_policy, trace_file):
num_blocks = cache_size // block_size
num_sets = num_blocks // associativity
cache = [[] for i in range(num_sets)]
hits = 0
misses = 0
with open(trace_file, "r") as f:
for line in f:
address = int(line.strip(), 16)
block_index = (address // block_size) % num_blocks
set_index = block_index // associativity
block = cache[set_index]
if block and block[0] == block_index:
hits += 1
if replacement_policy == "LRU":
block.remove(block_index)
block.append(block_index)
else:
misses += 1
if len(block) == associativity:
if replacement_policy == "LRU":
cache[set_index] = cache[set_index][1:]
cache[set_index].append(block_index)
elif replacement_policy == "FIFO":
cache[set_index] = cache[set_index][1:]
cache[set_index].append(block_index)
elif replacement_policy == "Random":
cache[set_index] = cache[set_index][1:]
cache[set_index].append(block_index)
else:
cache[set_index].append(block_index)
hit_rate = hits / (hits + misses)
return hit_rate
# Main function to run cache simulations
if __name__ == "__main__":
cache_size = int(input("Enter cache size (in bytes): "))
block_size = int(input("Enter block size (in bytes): "))
associativity = int(input("Enter associativity: "))
replacement_policy = input("Enter replacement policy (LRU/FIFO/Random): ")
trace_file = input("Enter path to trace file: ")
if cache_size not in sizes:
print("Invalid cache size")
sys.exit()
if block_size not in block_sizes:
print("Invalid block size")
sys.exit()
if associativity not in associativities:
print("Invalid associativity")
sys.exit()
if replacement_policy not in replacement_policies:
print("Invalid replacement policy")
sys.exit()
hit_rate = simulate_cache(cache_size, block_size, associativity, replacement_policy, trace_file)
print("Cache hit rate: {:.2f}%".format(hit_rate * 100))
```
您可以使用这个模拟器来模拟不同配置下的cache命中率,方法是输入cache大小、块大小、关联度和替换策略,以及trace文件的路径。模拟器将输出总的命中率。
需要注意的是,这个模拟器只能模拟单级cache,不支持多级cache。如果您需要模拟多级cache,需要对代码进行适当修改。
另外,这个模拟器只支持16进制的trace文件,如果您的trace文件是10进制的,需要将其转换为16进制。
相关推荐
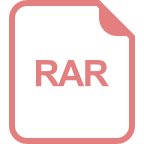
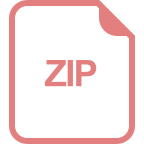
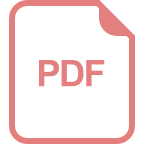












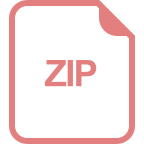
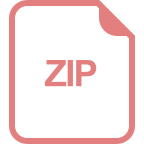
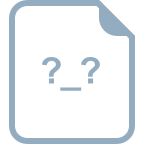