写一个c++代码满足银行账户管理系统设计与实现”,能够生成各类账户,配置基本属性并完成相关信息查询和显示。要求系统设计具有一定弹性和可扩展性,使得后续维护和扩展功能更容易,后续可以随时增加不同的账户类型
时间: 2023-06-13 08:07:40 浏览: 216
以下是一个简单的银行账户管理系统的C++代码,支持基本的账户类型,包括储蓄账户和支票账户。该代码基于面向对象的编程思想,具有一定的可扩展性和弹性,可以随时增加不同的账户类型。
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class Account {
public:
Account(string owner, double balance) {
m_owner = owner;
m_balance = balance;
}
virtual void display() {
cout << "Account owner: " << m_owner << endl;
cout << "Account balance: " << m_balance << endl;
}
virtual void deposit(double amount) {
m_balance += amount;
}
virtual void withdraw(double amount) {
if (m_balance >= amount) {
m_balance -= amount;
} else {
cout << "Insufficient balance" << endl;
}
}
protected:
string m_owner;
double m_balance;
};
class SavingsAccount : public Account {
public:
SavingsAccount(string owner, double balance, double interest_rate)
: Account(owner, balance), m_interest_rate(interest_rate) {}
virtual void display() {
cout << "Savings account" << endl;
Account::display();
cout << "Interest rate: " << m_interest_rate << endl;
}
private:
double m_interest_rate;
};
class CheckingAccount : public Account {
public:
CheckingAccount(string owner, double balance, double overdraft_limit)
: Account(owner, balance), m_overdraft_limit(overdraft_limit) {}
virtual void display() {
cout << "Checking account" << endl;
Account::display();
cout << "Overdraft limit: " << m_overdraft_limit << endl;
}
virtual void withdraw(double amount) {
if (m_balance + m_overdraft_limit >= amount) {
m_balance -= amount;
} else {
cout << "Exceeds overdraft limit" << endl;
}
}
private:
double m_overdraft_limit;
};
int main() {
vector<Account*> accounts;
Account* account1 = new SavingsAccount("Alice", 1000, 0.01);
accounts.push_back(account1);
Account* account2 = new CheckingAccount("Bob", 500, 1000);
accounts.push_back(account2);
for (int i = 0; i < accounts.size(); i++) {
accounts[i]->display();
}
account1->deposit(100);
account1->withdraw(200);
account1->display();
account2->withdraw(900);
account2->display();
return 0;
}
```
在该代码中,`Account` 是一个基类,包含了账户的基本属性和操作,包括账户所有者和余额、存款和取款等。`SavingsAccount` 和 `CheckingAccount` 是两个派生类,分别表示储蓄账户和支票账户。这两个派生类重写了基类的 `display` 和 `withdraw` 方法,以实现不同的功能。
在 `main` 函数中,我们创建了两个不同类型的账户,并将它们加入到 `accounts` 向量中。然后,我们遍历 `accounts` 向量,调用每个账户的 `display` 方法,以显示账户的信息。接着,我们对其中一个账户进行存款和取款操作,并再次显示账户信息。
该代码可以扩展添加新的账户类型,只需要创建一个新的派生类,并重写基类的方法。同时,我们使用了指针和向量等容器,以方便存储和管理不同类型的账户对象。
阅读全文
相关推荐
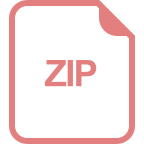
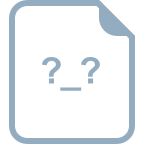
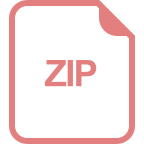

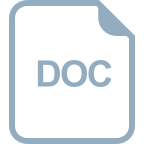
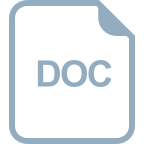
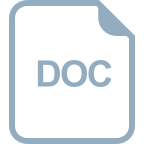
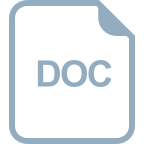
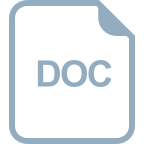


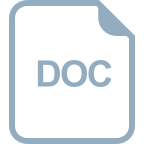
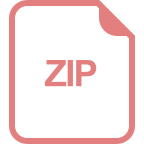
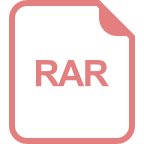
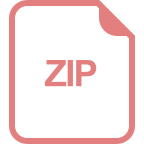
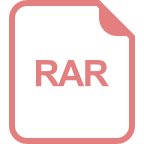
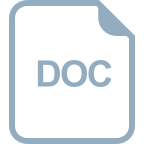
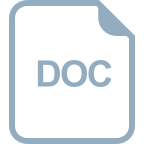
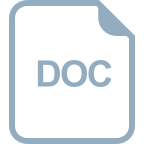