用c语言随机生成二元组序列
时间: 2024-11-05 13:08:04 浏览: 21
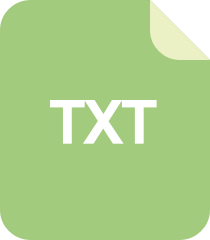
用C语言生成一个随机数独.txt
在C语言中,生成随机二元组序列通常涉及到两个步骤:首先生成随机数,然后用这些随机数构造二元组。这里我们可以使用标准库中的`<stdio.h>`和`<stdlib.h>`来完成这个任务。下面是一个简单的示例,用于生成指定长度的随机二元组:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// 定义二元组结构体
typedef struct {
int first;
int second;
} Tuple;
// 随机生成二元组函数
Tuple* generate_random_tuples(int length) {
// 设置随机种子以获得一致的结果 (可根据需要改变)
srand(time(0));
// 动态分配内存
Tuple* tuples = malloc(length * sizeof(Tuple));
if (!tuples) {
printf("Memory allocation failed.\n");
return NULL;
}
for (int i = 0; i < length; ++i) {
tuples[i].first = rand() % 100; // 生成0到99之间的随机整数
tuples[i].second = rand() % 100; // 同理,这里是第二个元素
}
return tuples;
}
// 打印二元组序列
void print_tuples(Tuple* tuples, int length) {
for (int i = 0; i < length; ++i) {
printf("(%d,%d)\n", tuples[i].first, tuples[i].second);
}
}
int main() {
int tuple_length = 5; // 想要生成的二元组数量
Tuple* random_tuples = generate_random_tuples(tuple_length);
if (random_tuples != NULL) {
print_tuples(random_tuples, tuple_length);
free(random_tuples); // 释放内存
} else {
return 1;
}
return 0;
}
```
阅读全文
相关推荐
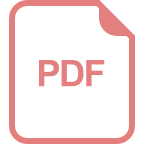
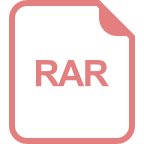
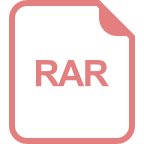
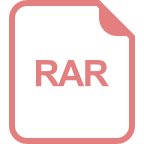
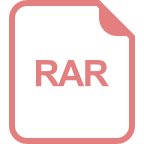
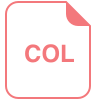
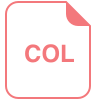
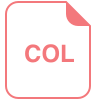


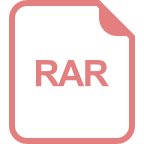
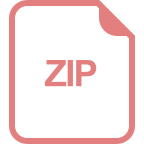
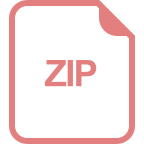
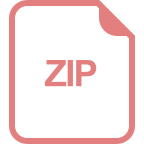
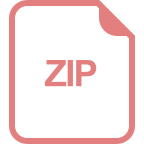