subprocess 实时获取输出,并依据输出内容进行输入
时间: 2023-12-16 22:04:00 浏览: 41
可以使用 Python 的 `subprocess` 模块来实现获取子进程的实时输出,并根据输出内容进行输入。
具体实现步骤如下:
1. 使用 `subprocess.Popen()` 函数启动子进程,并将 `stdout` 和 `stderr` 参数设置为 `subprocess.PIPE`,以便实时获取子进程的输出。
2. 使用 `p.stdout.readline()` 方法读取子进程的输出。该方法会阻塞,直到读取到一行输出或者子进程结束。
3. 根据读取到的输出内容,决定是否向子进程输入内容。可以使用 `p.stdin.write()` 方法向子进程输入内容。
4. 重复执行步骤 2 和步骤 3,直到子进程结束。
下面是一个示例代码,用于实现一个简单的与子进程交互的程序:
```python
import subprocess
# 启动子进程
p = subprocess.Popen(['my_program'], stdin=subprocess.PIPE, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
# 循环读取子进程的输出
while True:
output = p.stdout.readline().decode('utf-8')
if not output:
break
# 根据输出内容决定是否向子进程输入内容
if 'Enter your name:' in output:
p.stdin.write(b'My name is Alice\n')
p.stdin.flush()
# 等待子进程结束
p.wait()
```
需要注意的是,如果子进程的输出比较多,使用 `readline()` 方法阻塞读取输出可能会影响程序的性能。可以使用非阻塞的方式读取子进程的输出,例如使用 `select` 或者 `asyncio` 模块来实现。
相关推荐
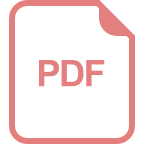
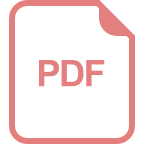
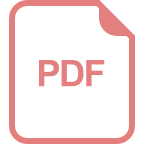














