javascipt练习//1统计一个字符串中a出现的次数 //2统计一个字符串中第一个字符出现的次数 //3统计一个字符串中每个字符出现的次数 //4统计一个字符串中出现次数最多的字符
时间: 2023-12-16 13:04:51 浏览: 76
1. 统计一个字符串中 a 出现的次数:
```javascript
function countA(str) {
let count = 0;
for (let i = 0; i < str.length; i++) {
if (str.charAt(i) === 'a') {
count++;
}
}
return count;
}
// 示例
console.log(countA('apple')); // 1
console.log(countA('banana')); // 3
```
2. 统计一个字符串中第一个字符出现的次数:
```javascript
function countFirstChar(str) {
let count = 0;
const firstChar = str.charAt(0);
for (let i = 0; i < str.length; i++) {
if (str.charAt(i) === firstChar) {
count++;
}
}
return count;
}
// 示例
console.log(countFirstChar('apple')); // 1
console.log(countFirstChar('banana')); // 2
```
3. 统计一个字符串中每个字符出现的次数:
```javascript
function countChars(str) {
const map = new Map();
for (let i = 0; i < str.length; i++) {
const char = str.charAt(i);
if (map.has(char)) {
map.set(char, map.get(char) + 1);
} else {
map.set(char, 1);
}
}
return map;
}
// 示例
console.log(countChars('apple'));
// Map(4) { 'a' => 1, 'p' => 2, 'l' => 1, 'e' => 1 }
console.log(countChars('banana'));
// Map(3) { 'b' => 1, 'a' => 3, 'n' => 2 }
```
4. 统计一个字符串中出现次数最多的字符:
```javascript
function mostFrequentChar(str) {
const map = new Map();
for (let i = 0; i < str.length; i++) {
const char = str.charAt(i);
if (map.has(char)) {
map.set(char, map.get(char) + 1);
} else {
map.set(char, 1);
}
}
let maxCount = 0;
let maxChar = '';
for (const [char, count] of map.entries()) {
if (count > maxCount) {
maxCount = count;
maxChar = char;
}
}
return maxChar;
}
// 示例
console.log(mostFrequentChar('apple')); // 'p'
console.log(mostFrequentChar('banana')); // 'a'
```
阅读全文
相关推荐
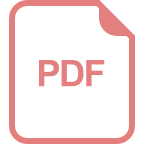
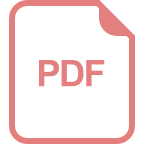
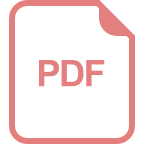















