用c语言1.设计一个“图形”类shape,基类部分的共同特征(如宽等)和方法(如初始化、求面积等)。以此为基础,派生出矩形rectangle、圆形circle。二者都有计算面积的函数以及计算周长的函数。并编写相应主函数进行测试。
时间: 2023-02-16 20:43:55 浏览: 71
下面是示例代码:
```c
#include <stdio.h>
// 定义基类 "图形"
typedef struct {
int width;
int height;
} Shape;
// 初始化函数
void shape_init(Shape* shape, int width, int height) {
shape->width = width;
shape->height = height;
}
// 求面积函数
int shape_area(Shape* shape) {
return shape->width * shape->height;
}
// 定义派生类 "矩形"
typedef struct {
Shape shape;
// 可以在此处添加其他成员
} Rectangle;
// 初始化函数
void rectangle_init(Rectangle* rect, int width, int height) {
shape_init((Shape*)rect, width, height);
}
// 求面积函数
int rectangle_area(Rectangle* rect) {
return shape_area((Shape*)rect);
}
// 求周长函数
int rectangle_perimeter(Rectangle* rect) {
return 2 * (rect->shape.width + rect->shape.height);
}
// 定义派生类 "圆形"
typedef struct {
Shape shape;
// 可以在此处添加其他成员
} Circle;
// 初始化函数
void circle_init(Circle* circle, int radius) {
shape_init((Shape*)circle, radius, radius);
}
// 求面积函数
int circle_area(Circle* circle) {
return shape_area((Shape*)circle) * 3.14159;
}
// 求周长函数
int circle_perimeter(Circle* circle) {
return 2 * 3.14159 * circle->shape.width;
}
int main() {
Rectangle rect;
rectangle_init(&rect, 10, 5);
printf("矩形的面积是 %d\n", rectangle_area(&rect));
printf("矩形的周长是 %d\n", rectangle_perimeter(&rect));
Circle circle;
circle_init(&circle, 5);
printf("圆形的面积是 %d\n", circle_area(&circle));
printf("圆形的周长是 %d\n", circle_perimeter(&circle));
return 0;
}
```
希望这个示例代码能帮助到你。
相关推荐
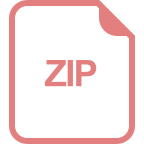
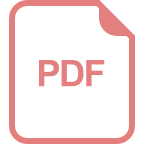












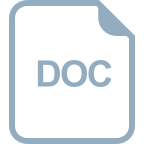
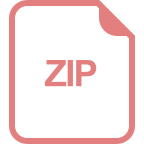
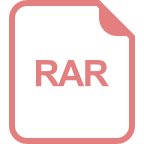