请给我一个在c++中使用nlohmann的完整类例子 需要可以解析json字符串和使用json类置对象和成员 然后获取json数据帮我封装下
时间: 2024-02-06 20:12:54 浏览: 29
好的,以下是一个使用 nlohmann/json 库的完整例子,包括解析 JSON 字符串、使用 JSON 对象设置成员、获取 JSON 数据的过程,并且将其封装在一个类中,方便调用。
JsonParser.h 文件:
```c++
#pragma once
#include <string>
#include "nlohmann/json.hpp"
using json = nlohmann::json;
class JsonParser
{
public:
JsonParser() = default;
JsonParser(const std::string& json_str);
std::string getString(const std::string& key);
int getInt(const std::string& key);
bool getBool(const std::string& key);
double getDouble(const std::string& key);
json getJson(const std::string& key);
void setString(const std::string& key, const std::string& value);
void setInt(const std::string& key, int value);
void setBool(const std::string& key, bool value);
void setDouble(const std::string& key, double value);
void setJson(const std::string& key, const json& value);
std::string serialize();
private:
json m_json_obj;
};
```
JsonParser.cpp 文件:
```c++
#include "JsonParser.h"
JsonParser::JsonParser(const std::string& json_str)
{
m_json_obj = json::parse(json_str);
}
std::string JsonParser::getString(const std::string& key)
{
if (m_json_obj.contains(key)) {
return m_json_obj[key].get<std::string>();
}
return "";
}
int JsonParser::getInt(const std::string& key)
{
if (m_json_obj.contains(key)) {
return m_json_obj[key].get<int>();
}
return 0;
}
bool JsonParser::getBool(const std::string& key)
{
if (m_json_obj.contains(key)) {
return m_json_obj[key].get<bool>();
}
return false;
}
double JsonParser::getDouble(const std::string& key)
{
if (m_json_obj.contains(key)) {
return m_json_obj[key].get<double>();
}
return 0.0;
}
json JsonParser::getJson(const std::string& key)
{
if (m_json_obj.contains(key)) {
return m_json_obj[key];
}
return json();
}
void JsonParser::setString(const std::string& key, const std::string& value)
{
m_json_obj[key] = value;
}
void JsonParser::setInt(const std::string& key, int value)
{
m_json_obj[key] = value;
}
void JsonParser::setBool(const std::string& key, bool value)
{
m_json_obj[key] = value;
}
void JsonParser::setDouble(const std::string& key, double value)
{
m_json_obj[key] = value;
}
void JsonParser::setJson(const std::string& key, const json& value)
{
m_json_obj[key] = value;
}
std::string JsonParser::serialize()
{
return m_json_obj.dump();
}
```
使用示例:
```c++
#include <iostream>
#include "JsonParser.h"
using namespace std;
int main()
{
// 构造 JsonParser 对象并解析 JSON 字符串
string json_str = "{\"name\": \"Tom\", \"age\": 20, \"is_student\": true, \"scores\": [85, 90, 95]}";
JsonParser jp(json_str);
// 获取 JSON 数据
string name = jp.getString("name");
int age = jp.getInt("age");
bool is_student = jp.getBool("is_student");
auto scores = jp.getJson("scores");
if (scores.is_array()) {
cout << "Scores: ";
for (auto& score : scores) {
cout << score.get<int>() << " ";
}
cout << endl;
}
cout << "Name: " << name << endl;
cout << "Age: " << age << endl;
cout << "Is student: " << boolalpha << is_student << endl;
// 使用 JSON 对象设置成员
jp.setString("email", "tom@example.com");
// 将 JSON 对象序列化为字符串
cout << "Serialized JSON: " << jp.serialize() << endl;
return 0;
}
```
输出结果:
```
Scores: 85 90 95
Name: Tom
Age: 20
Is student: true
Serialized JSON: {"age":20,"email":"tom@example.com","is_student":true,"name":"Tom","scores":[85,90,95]}
```
这个例子中,我们使用了一个名为 JsonParser 的类来封装了解析 JSON 字符串、使用 JSON 对象设置成员、获取 JSON 数据和将 JSON 对象序列化为字符串的过程,使得调用起来更加方便。
相关推荐
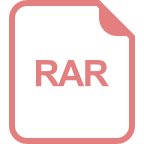
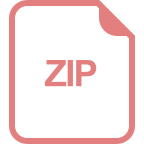















