#include <stdio.h> #include <math.h> // 待求极值的函数 double f(double x) { return pow(x, 3) - 2 * pow(x, 2) + 3 * x - 1; } // 求函数在x处的导数 double df(double x, double h) { return (f(x + h) - f(x - h)) / (2 * h); } // 求函数的极值 double find_extremum(double a, double b, double eps) { double x0, x1, x2, h; x1 = a; x2 = b; h = 0.001; do { x0 = x1; x1 = x2; x2 = x1 - df(x1, h) / ((df(x1, h) - df(x0, h)) / (x1 - x0)); } while (fabs(x2 - x1) >= eps); return x2; } int main() { double a, b, eps; printf("请输入区间[a, b]和精度eps:\n"); scanf("%lf %lf %lf", &a, &b, &eps); double x = find_extremum(a, b, eps); printf("函数的极值点为:%lf\n", x); printf("函数的极值为:%lf\n", f(x)); return 0; }加注释
时间: 2023-12-10 14:04:12 浏览: 70
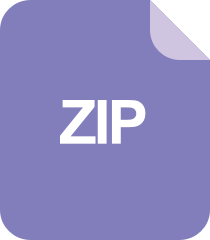
SeqList.h #include <stdio.h> //包含标准输入输出流的头文件 #include <assert.h
```c
#include <stdio.h>
#include <math.h>
// 待求极值的函数
double f(double x) {
return pow(x, 3) - 2 * pow(x, 2) + 3 * x - 1;
}
// 求函数在x处的导数
double df(double x, double h) {
return (f(x + h) - f(x - h)) / (2 * h);
}
// 求函数的极值
double find_extremum(double a, double b, double eps) {
double x0, x1, x2, h;
x1 = a;
x2 = b;
h = 0.001;
do {
x0 = x1;
x1 = x2;
// 使用牛顿迭代法求解极值点
x2 = x1 - df(x1, h) / ((df(x1, h) - df(x0, h)) / (x1 - x0));
} while (fabs(x2 - x1) >= eps);
return x2;
}
int main() {
double a, b, eps;
// 获取区间和精度
printf("请输入区间[a, b]和精度eps:\n");
scanf("%lf %lf %lf", &a, &b, &eps);
// 调用函数求解
double x = find_extremum(a, b, eps);
printf("函数的极值点为:%lf\n", x);
printf("函数的极值为:%lf\n", f(x));
return 0;
}
```
阅读全文
相关推荐
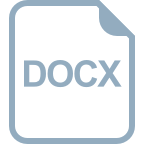
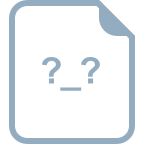















