qgraphicsitem设置不可移动
时间: 2024-03-27 09:35:57 浏览: 87
要设置QGraphicsItem不可移动,可以通过重写mouseMoveEvent()函数并将其禁用来实现。下面是一个示例:
```python
class MyGraphicsItem(QGraphicsItem):
def __init__(self):
super().__init__()
def mouseMoveEvent(self, event):
# 禁用父类中的mouseMoveEvent()函数
pass
```
这样,当你尝试移动该图形项时,它将不会发生任何变化。当然,你还可以在此基础上添加其他的交互操作。
相关问题
使用代码实现 定义棋子的移动方式,车的走棋规则为:横着走或竖着走,不可斜着走,且不能越过其他棋子。
好的,以下是一个简单的代码实现:
```cpp
// 定义 ChessPiece 类,继承自 QGraphicsItem
class ChessPiece : public QGraphicsItem {
public:
// 构造函数,传入棋子类型和所在位置
ChessPiece(QPoint pos, ChessType type) : m_pos(pos), m_type(type) {}
// 重写 boundingRect() 函数,返回棋子的矩形区域
QRectF boundingRect() const override {
return QRectF(-45, -45, 90, 90);
}
// 重写 paint() 函数,绘制棋子
void paint(QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget) override {
// 根据棋子类型和位置绘制不同的图案
if (m_type == ChessType::CHE) {
painter->drawText(QPointF(0, 0), "车");
}
// ...
}
// 重写 mousePressEvent() 函数,响应鼠标点击事件
void mousePressEvent(QGraphicsSceneMouseEvent *event) override {
// 如果当前棋子可以移动,计算出所有可移动的位置
if (canMove()) {
QList<QPoint> moveList = getMoveList();
// 在棋盘上将可移动的位置用圆圈标出
for (const auto &p : moveList) {
QGraphicsEllipseItem *ellipse = new QGraphicsEllipseItem(QRectF(p.x() * 90 - 15, p.y() * 90 - 15, 30, 30));
ellipse->setPen(Qt::NoPen);
ellipse->setBrush(Qt::red);
scene()->addItem(ellipse);
}
}
}
private:
QPoint m_pos; // 棋子坐标
ChessType m_type; // 棋子类型
// 判断当前棋子是否可以移动
bool canMove() {
// 获取棋盘上所有的棋子
QList<QGraphicsItem *> items = scene()->items();
for (const auto &item : items) {
ChessPiece *piece = dynamic_cast<ChessPiece *>(item);
if (piece && piece->pos() != m_pos) { // 如果不是当前棋子,并且不在当前位置
// 如果目标位置与当前位置的横坐标或纵坐标相等,且之间没有其他棋子,则可以移动
if ((piece->pos().x() == m_pos.x() && qAbs(piece->pos().y() - m_pos.y()) < 90)
|| (piece->pos().y() == m_pos.y() && qAbs(piece->pos().x() - m_pos.x()) < 90)) {
return false;
}
}
}
return true;
}
// 获取当前棋子可以移动的位置列表
QList<QPoint> getMoveList() {
QList<QPoint> moveList;
for (int i = 0; i < 9; i++) {
if (i != m_pos.x() / 90) { // 如果不是当前列
moveList.append(QPoint(i, m_pos.y() / 90));
}
if (i != m_pos.y() / 90) { // 如果不是当前行
moveList.append(QPoint(m_pos.x() / 90, i));
}
}
return moveList;
}
};
```
上述代码中,canMove() 函数用来判断当前棋子是否可以移动,getMoveList() 函数用来获取当前棋子可以移动的位置列表。在 mousePressEvent() 函数中,如果当前棋子可以移动,就计算出所有可移动的位置,并在棋盘上将可移动的位置用圆圈标出。当然,这只是一个简单的实现,实际情况可能更复杂,还需要根据具体的需求进行调整。
AttributeError: 'QGraphicsView' object has no attribute 'setFlag'
根据提供的引用内容,第一个引用说明了在QGraphicsItem类中有一个函数叫做setHandlesChildEvents,它用于设置是否处理子事件。第二个引用和第三个引用是关于setFlags函数的使用的示例。这个函数用于设置QGraphicsItem对象的标志,比如是否可选择和是否可移动。
根据你提供的错误信息"AttributeError: 'QGraphicsView' object has no attribute 'setFlag'",它表示QGraphicsView对象没有setFlag这个属性。这个错误通常是因为你尝试在QGraphicsView对象上调用了setFlag函数,而实际上这个函数是属于QGraphicsItem类的,不是属于QGraphicsView类的。
所以,解决这个错误的方法是确保你在正确的对象上调用正确的函数。在这种情况下,你可能需要检查你的代码,确认你是在QGraphicsItem对象上调用setFlag函数,而不是在QGraphicsView对象上调用。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [qt blog](https://blog.csdn.net/qq_33564134/article/details/80450321)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
阅读全文
相关推荐
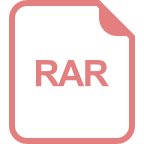
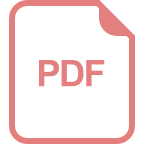
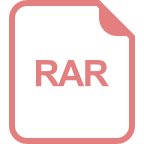
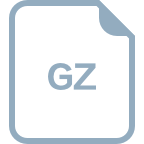
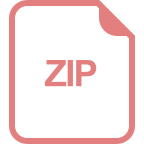
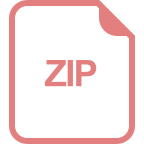
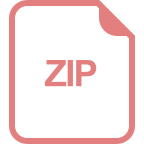
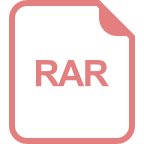
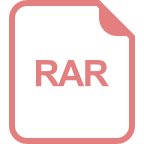
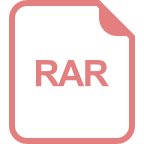
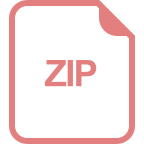
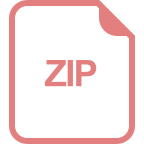
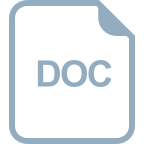
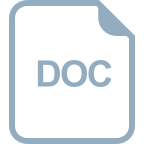
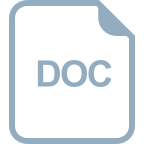

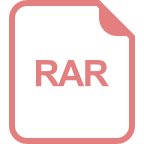