implement quick sort by C
时间: 2024-05-19 12:15:15 浏览: 99
Sure, here's an example implementation of quick sort in C:
void quicksort(int array[], int left, int right) {
if(left < right) {
int pivot = partition(array, left, right);
quicksort(array, left, pivot - 1);
quicksort(array, pivot + 1, right);
}
}
int partition(int array[], int left, int right) {
int pivot = array[right];
int i = left - 1;
for(int j = left; j < right; j++) {
if(array[j] < pivot) {
i++;
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
}
int temp = array[i + 1];
array[i + 1] = array[right];
array[right] = temp;
return i + 1;
}
Hope that helps!
相关问题
Try to write an algorithm to implement quick sort.
Sure, here is a simple algorithm to implement Quick Sort:
1. Choose a pivot element, which can be any element from the array.
2. Partition the array around the pivot element, such that all the elements to the left of the pivot are smaller than the pivot element, and all the elements to the right of the pivot are greater than or equal to the pivot element.
3. Recursively apply this process to the sub-arrays to the left and right of the pivot until the entire array is sorted.
Here is the step-by-step implementation of the algorithm in pseudocode:
```
function quicksort(array)
if length(array) <= 1
return array
else
pivot = choose_pivot(array)
left = []
right = []
for element in array
if element < pivot
append element to left
else if element >= pivot
append element to right
sorted_left = quicksort(left)
sorted_right = quicksort(right)
return concatenate(sorted_left, [pivot], sorted_right)
```
In this implementation, the `choose_pivot` function can be any function to select a pivot element. A common approach is to select the first or last element of the array as the pivot, or to select a random element.
Try to write an algorithm to implement quick sort.加上注释
好的,下面是带注释的快速排序算法实现,注释会解释每个步骤的作用。
```
# 定义快速排序函数,输入为待排序的数组
def quick_sort(arr):
# 如果数组长度小于等于1,直接返回数组
if len(arr) <= 1:
return arr
else:
# 选择一个基准元素,可以是任意一个元素,此处选择数组中间的元素
pivot = arr[len(arr) // 2]
# 定义左、右两个空数组,用于存放比基准元素小的元素和比基准元素大的元素
left = []
right = []
# 遍历数组中的每一个元素,将其与基准元素进行比较,并将其放入左、右数组中
for item in arr:
if item < pivot:
left.append(item)
else:
right.append(item)
# 递归调用快速排序函数,对左、右两个数组进行排序
left = quick_sort(left)
right = quick_sort(right)
# 将左、右两个数组和基准元素合并起来,返回排好序的数组
return left + [pivot] + right
```
在这个实现中,基准元素的选择可以是任意一个元素,此处选择了数组中间的元素。在实际应用中,基准元素的选择会影响算法的效率,通常采用随机选择、三数取中等方法来减少不平衡情况的发生。
相关推荐
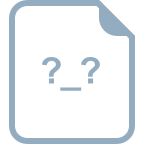












