implement gpio lighting的c代码
时间: 2023-06-13 14:04:00 浏览: 138
以下是一个简单的 GPIO 控制 LED 灯的 C 代码,使用 Raspberry Pi 作为例子:
```c
#include <stdio.h>
#include <wiringPi.h>
#define LED_PIN 17 // GPIO 17,对应物理引脚11
int main(void) {
if (wiringPiSetupGpio() == -1) { // 初始化 GPIO 库
printf("Failed to setup GPIO.\n");
return 1;
}
pinMode(LED_PIN, OUTPUT); // 设置引脚为输出模式
while (1) {
digitalWrite(LED_PIN, HIGH); // 开灯
delay(1000); // 延时1秒
digitalWrite(LED_PIN, LOW); // 关灯
delay(1000); // 延时1秒
}
return 0;
}
```
需要注意的是,这个程序需要以 root 权限运行,因为需要访问硬件资源。可以使用以下命令编译和运行程序:
```bash
$ gcc -o gpio_led gpio_led.c -lwiringPi
$ sudo ./gpio_led
```
相关问题
俄罗斯方块初始界面c语言代码
要创建一个基本的俄罗斯方块游戏的初始界面,我们需要考虑以下几个关键元素:窗口初始化、标题设置、以及基本的图形绘制。由于你是请求用C语言编写,这里我会提供一个简单的控制台版本的示例,因为使用C库如ncurses或SDL在Windows上可能更复杂。
```c
#include <stdio.h>
#include <conio.h> // Windows console input functions
// Function prototypes
void draw_board(int board[4][6]); // Draw the game board
void display_message(char *msg); // Display a message
int main() {
int board[4][6] = {0}; // Initialize an empty board
char msg[50] = "Welcome to Tetris! Press any key to start..."; // Initial message
bool playing = false; // Flag to indicate if game is running
while (true) {
// Check for user input to start the game or exit
if (_kbhit()) {
playing = true;
break;
}
display_message(msg);
draw_board(board);
}
if (playing) {
printf("Starting game...\n");
// Game logic goes here, which you'll need to implement separately
} else {
printf("Exiting...\n");
}
return 0;
}
// Draw the board function, this is a placeholder and would need to be expanded
void draw_board(int board[4][6]) {
for (int i = 0; i < 4; ++i) {
for (int j = 0; j < 6; ++j) {
// You can use ASCII characters to represent blocks on the board
printf("%c ", board[i][j]);
}
printf("\n");
}
}
// Display a message function, also a placeholder
void display_message(char *msg) {
printf("%s\n", msg);
}
```
这个代码只是一个起点,实际的游戏逻辑(例如旋转、下落和消除行)需要你自己去实现。而且,为了创建一个完整的图形化的界面,你可能需要使用像`ncurses`这样的库,而不是标准的`printf`和`_kbhit()`函数。
快速排序c语言代码以及解释
Sure, here's an example of the Quick Sort algorithm implemented in C:
```c
#include<stdio.h>
// Function to swap two elements
void swap(int* a, int* b) {
int temp = *a;
*a = *b;
*b = temp;
}
// Partition the array on the basis of pivot
int partition(int arr[], int low, int high) {
int pivot = arr[high];
int i = (low - 1);
for (int j = low; j <= high - 1; j++) {
if (arr[j] < pivot) {
i++;
swap(&arr[i], &arr[j]);
}
}
swap(&arr[i + 1], &arr[high]);
return (i + 1);
}
// Function to implement Quick Sort
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
// Function to print the array
void printArray(int arr[], int size) {
for (int i = 0; i < size; i++) {
printf("%d ", arr[i]);
}
printf("\n");
}
// Driver program to test above functions
int main() {
int arr[] = {10, 7, 8, 9, 1, 5};
int n = sizeof(arr) / sizeof(arr[0]);
printf("Original array:\n");
printArray(arr, n);
quickSort(arr, 0, n - 1);
printf("Sorted array:\n");
printArray(arr, n);
return 0;
}
```
Explanation:
1. The `swap` function swaps the values of two elements in the array.
2. The `partition` function chooses a pivot element (in this implementation, the last element) and rearranges the array such that all elements smaller than the pivot are on its left and all elements greater than the pivot are on its right. It returns the index of the pivot element.
3. The `quickSort` function recursively calls itself to sort the subarrays before and after the partition.
4. The `printArray` function is used to print the elements of the array.
5. In the `main` function, an array is defined, its original state is printed, and then `quickSort` is called to sort the array. Finally, the sorted array is printed.
This implementation of Quick Sort has a time complexity of O(n log n) in average and best cases, and O(n^2) in the worst case.
阅读全文
相关推荐
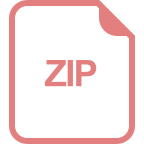
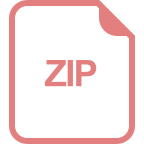













