请给出生成word文档最简单的前后端springboot代码实现
时间: 2023-06-11 16:06:37 浏览: 48
这里提供一个简单的前后端分离的Spring Boot项目,实现了生成Word文档的功能。
后端代码(使用Apache POI实现):
1.在pom.xml中添加以下依赖:
```xml
<dependencies>
<!-- Spring Boot Web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Apache POI -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.2</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.2</version>
</dependency>
</dependencies>
```
2.在Controller中添加生成Word文档的接口:
```java
@RestController
public class WordController {
@GetMapping("/word")
public ResponseEntity<Resource> generateWord() throws IOException {
// 生成Word文档
XWPFDocument document = new XWPFDocument();
XWPFParagraph paragraph = document.createParagraph();
XWPFRun run = paragraph.createRun();
run.setText("Hello, World!");
// 将生成的Word文档保存到临时文件中
File tempFile = File.createTempFile("temp", ".docx");
FileOutputStream outputStream = new FileOutputStream(tempFile);
document.write(outputStream);
outputStream.close();
// 返回Word文档作为文件下载
FileSystemResource file = new FileSystemResource(tempFile);
HttpHeaders headers = new HttpHeaders();
headers.add(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + file.getFilename() + "\"");
return ResponseEntity.ok()
.headers(headers)
.contentLength(file.contentLength())
.contentType(MediaType.APPLICATION_OCTET_STREAM)
.body(file);
}
}
```
前端代码:
1.在HTML页面中添加“生成Word文档”的按钮:
```html
<button onclick="generateWord()">生成Word文档</button>
```
2.在JavaScript中实现生成Word文档的功能:
```javascript
function generateWord() {
// 发送GET请求
var xhr = new XMLHttpRequest();
xhr.open('GET', '/word', true);
xhr.responseType = 'blob';
// 处理响应结果
xhr.onload = function() {
if (this.status === 200) {
var blob = new Blob([this.response], { type: 'application/vnd.openxmlformats-officedocument.wordprocessingml.document' });
var link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = 'example.docx';
link.click();
}
};
xhr.send();
}
```
以上代码实现了在点击“生成Word文档”按钮时,向后端发送GET请求并下载生成的Word文档。注意,这里使用了XMLHttpRequest对象发送GET请求,并使用Blob对象处理响应结果。同时,由于下载的是Word文档,需要设置blob的类型为“application/vnd.openxmlformats-officedocument.wordprocessingml.document”。
相关推荐
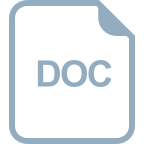
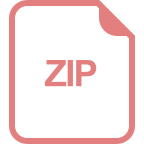
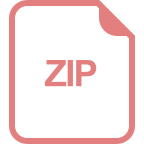









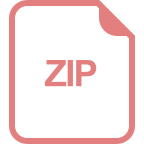
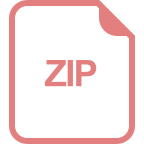
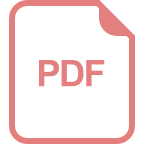
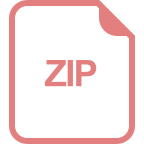