delphi 485 代码
时间: 2023-09-29 17:03:02 浏览: 38
以下是一个简单的 Delphi 代码示例,用于通过 RS485 协议进行通信:
```
unit Unit1;
interface
uses
Windows, Messages, SysUtils, Variants, Classes, Graphics, Controls, Forms,
Dialogs, StdCtrls;
const
// 串口号
COM_PORT = 'COM1';
// 波特率
BAUD_RATE = 9600;
// 数据位
DATA_BITS = 8;
// 停止位
STOP_BITS = 1;
// 校验位
PARITY = NOPARITY;
type
TForm1 = class(TForm)
Memo1: TMemo;
Edit1: TEdit;
Button1: TButton;
procedure Button1Click(Sender: TObject);
procedure FormCreate(Sender: TObject);
private
{ Private declarations }
FComHandle: THandle;
procedure WriteData(Data: AnsiString);
function ReadData: AnsiString;
public
{ Public declarations }
end;
var
Form1: TForm1;
implementation
{$R *.dfm}
// 打开串口
function OpenCom(Port: string; BaudRate: Integer; DataBits: Integer; StopBits: Integer; Parity: Integer): THandle;
var
DCB: TDCB;
PortHandle: THandle;
begin
Result := INVALID_HANDLE_VALUE;
PortHandle := CreateFile(PChar(Port), GENERIC_READ or GENERIC_WRITE, 0, nil, OPEN_EXISTING, 0, 0);
if PortHandle = INVALID_HANDLE_VALUE then Exit;
FillChar(DCB, SizeOf(DCB), 0);
DCB.DCBlength := SizeOf(DCB);
if not GetCommState(PortHandle, DCB) then Exit;
DCB.BaudRate := BaudRate;
DCB.ByteSize := DataBits;
DCB.Parity := Parity;
DCB.StopBits := StopBits;
if not SetCommState(PortHandle, DCB) then Exit;
Result := PortHandle;
end;
// 关闭串口
procedure CloseCom(Handle: THandle);
begin
CloseHandle(Handle);
end;
// 写入数据
procedure TForm1.WriteData(Data: AnsiString);
var
BytesWritten: DWORD;
begin
if WriteFile(FComHandle, Data[1], Length(Data), BytesWritten, nil) then
begin
Memo1.Lines.Add('Write: ' + Data);
end
else
begin
Memo1.Lines.Add('Error writing to port');
end;
end;
// 读取数据
function TForm1.ReadData: AnsiString;
var
Buffer: array[0..1024] of AnsiChar;
BytesRead: DWORD;
i, j: Integer;
begin
Result := '';
if ReadFile(FComHandle, Buffer, SizeOf(Buffer), BytesRead, nil) then
begin
for i := 0 to BytesRead - 1 do
begin
if (Buffer[i] = #13) or (Buffer[i] = #10) then
begin
for j := 0 to i - 1 do
begin
Result := Result + Buffer[j];
end;
Exit;
end;
end;
end
else
begin
Memo1.Lines.Add('Error reading from port');
end;
end;
procedure TForm1.FormCreate(Sender: TObject);
begin
FComHandle := OpenCom(COM_PORT, BAUD_RATE, DATA_BITS, STOP_BITS, PARITY);
if FComHandle = INVALID_HANDLE_VALUE then
begin
ShowMessage('Failed to open serial port');
end;
end;
procedure TForm1.Button1Click(Sender: TObject);
begin
WriteData(Edit1.Text + #13#10);
Memo1.Lines.Add('Read: ' + ReadData);
end;
// 关闭串口
procedure TForm1.FormClose(Sender: TObject; var Action: TCloseAction);
begin
CloseCom(FComHandle);
end;
end.
```
在这个示例中,我们使用 Windows API 来打开和关闭串口,使用 `WriteFile` 函数向串口写入数据,并使用 `ReadFile` 函数从串口读取数据。请注意,这个示例仅仅是一个简单的示例,你需要根据你的具体需求修改代码。
相关推荐
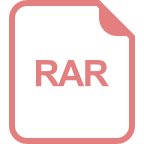

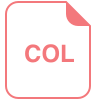












