提供Pinus 客户端库 代码
时间: 2023-06-25 15:02:55 浏览: 98
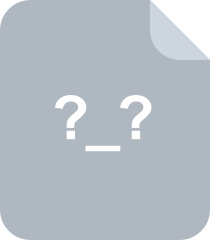
客户端代码
Pinus 客户端库有多种语言的实现,以下是其中几种常见语言的代码示例:
### JavaScript
```javascript
const pinus = require('pinus');
const client = pinus.createClient({
host: '127.0.0.1',
port: 3014
});
client.on('onChat', function(data) {
console.log(data);
});
client.request('chat.chatHandler.send', {content: 'hello world!'}, function(err, data) {
if (err) {
console.error(err);
return;
}
console.log(data);
});
```
### Java
```java
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.SimpleChannelInboundHandler;
import io.netty.util.AttributeKey;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class PinusClientHandler extends SimpleChannelInboundHandler<String> {
private static final Logger logger = LoggerFactory.getLogger(PinusClientHandler.class);
private PinusClient client;
public PinusClientHandler(PinusClient client) {
this.client = client;
}
@Override
protected void channelRead0(ChannelHandlerContext ctx, String msg) throws Exception {
logger.debug("Received message from server: {}", msg);
JSONObject json = JSON.parseObject(msg);
String route = json.getString("route");
JSONObject body = json.getJSONObject("body");
if (route == null || body == null) {
return;
}
if ("onChat".equals(route)) {
String content = body.getString("content");
String from = body.getString("from");
client.onChat(from, content);
}
}
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
logger.debug("Channel active.");
ctx.channel().attr(AttributeKey.valueOf("pinusClient")).set(client);
client.connectSuccess();
}
@Override
public void channelInactive(ChannelHandlerContext ctx) throws Exception {
logger.debug("Channel inactive.");
client.connectClosed();
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
logger.error("Exception caught.", cause);
client.connectError(cause);
}
}
```
### Python
```python
from socketIO_client import SocketIO, BaseNamespace
class PinusClient(BaseNamespace):
def on_connect(self):
print('Connected to server.')
def on_disconnect(self):
print('Disconnected from server.')
def on_chat(self, data):
print('Received message: ', data)
client = PinusClient('/chat')
socketIO = SocketIO('127.0.0.1', 3014)
socketIO.define(client)
socketIO.wait()
```
以上代码仅供参考,具体实现需要根据自己的需求进行相应修改。
阅读全文
相关推荐



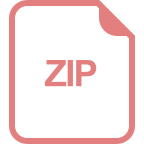







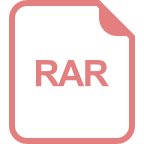
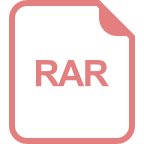
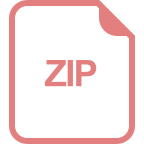
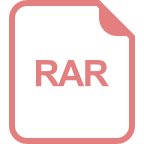
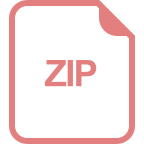