C++语言来获取当前路径的文件目录
时间: 2023-05-26 21:04:12 浏览: 106
在 C 语言中,可以使用以下方法获取当前路径的文件目录:
```c
#include <stdio.h>
#include <unistd.h>
int main() {
char cwd[1024];
if (getcwd(cwd, sizeof(cwd)) != NULL) {
printf("当前路径:%s\n", cwd);
} else {
perror("getcwd() 错误");
return 1;
}
return 0;
}
```
这段代码使用了 `getcwd()` 函数来获取当前工作目录,并将其存储在一个名为 `cwd` 的字符数组中。如果获取成功,该数组中将包含当前路径的文件目录,否则将打印出一条错误消息。
相关问题
NX二次开发C++ 获取dll所在文件路径
您可以使用以下方法来获取 DLL 文件所在的路径:
1. 首先,使用 `GetModuleHandle` 函数获取 DLL 入口点的句柄。这个函数的参数是 DLL 的文件名或者路径,如果传入 NULL,则返回当前进程的句柄。
```cpp
HMODULE hModule = GetModuleHandle(NULL);
```
2. 然后,使用 `GetModuleFileName` 函数来获取 DLL 文件的路径。这个函数的参数是 DLL 的句柄和一个缓冲区,用于存储文件路径。
```cpp
char path[MAX_PATH];
GetModuleFileName(hModule, path, MAX_PATH);
```
3. 最后,您可以将获取到的路径转换为 `std::string` 类型,以便更方便地使用。
```cpp
std::string dllPath(path);
```
这样,您就可以通过 `dllPath` 变量获取到 DLL 文件所在的路径了。请注意,以上代码是在 Windows 平台下使用 C++ 编写的。如果您是在其他平台或使用其他编程语言,请适当修改代码。
基于VS2022用C++语言编写一个删除Windows系统文件资源管理器文件检索记录的程序
首先,我们需要了解Windows系统文件资源管理器的文件检索记录存储在哪里。在Windows 10中,这些记录存储在以下路径下的一个名为“Recent”(最近)的文件夹中:
```
C:\Users\<用户名>\AppData\Roaming\Microsoft\Windows\Recent
```
接下来,我们需要编写一个程序来删除这些记录。程序的基本思路是打开这个文件夹,逐个删除其中的文件。
以下是一个基于VS2022和C++的示例代码:
```c++
#include <iostream>
#include <windows.h>
using namespace std;
int main()
{
// 获取用户目录路径
TCHAR szUserPath[MAX_PATH];
if (!SUCCEEDED(SHGetFolderPath(NULL, CSIDL_PROFILE, NULL, 0, szUserPath)))
{
cout << "获取用户目录路径失败!" << endl;
return 1;
}
// 拼接文件夹路径
TCHAR szRecentPath[MAX_PATH];
if (!SUCCEEDED(PathCombine(szRecentPath, szUserPath, _T("AppData\\Roaming\\Microsoft\\Windows\\Recent"))))
{
cout << "拼接文件夹路径失败!" << endl;
return 1;
}
// 打开文件夹
HANDLE hFind;
WIN32_FIND_DATA fd;
TCHAR szFindPath[MAX_PATH];
if (!SUCCEEDED(PathCombine(szFindPath, szRecentPath, _T("*"))))
{
cout << "拼接查找路径失败!" << endl;
return 1;
}
hFind = FindFirstFile(szFindPath, &fd);
if (hFind == INVALID_HANDLE_VALUE)
{
cout << "打开文件夹失败!" << endl;
return 1;
}
// 遍历文件夹中的文件,并删除
do
{
if (!(fd.dwFileAttributes & FILE_ATTRIBUTE_DIRECTORY))
{
TCHAR szFilePath[MAX_PATH];
if (!SUCCEEDED(PathCombine(szFilePath, szRecentPath, fd.cFileName)))
{
cout << "拼接文件路径失败!" << endl;
continue;
}
if (!DeleteFile(szFilePath))
{
cout << "删除文件失败:" << szFilePath << endl;
}
else
{
cout << "删除文件成功:" << szFilePath << endl;
}
}
} while (FindNextFile(hFind, &fd));
// 关闭文件夹句柄
FindClose(hFind);
cout << "文件检索记录已删除!" << endl;
return 0;
}
```
这个程序使用了Windows API来获取用户目录路径、拼接文件夹路径、打开文件夹、遍历文件夹中的文件并删除。在遍历文件夹时,我们只删除文件,不删除子文件夹。在删除文件时,我们使用了DeleteFile函数。
注意,这个程序需要管理员权限才能成功删除文件检索记录。你可以在程序中添加代码来检查当前用户是否是管理员,并提示用户需要以管理员身份运行程序。
另外,删除文件检索记录可能会影响到Windows系统的一些功能,比如最近打开的文件列表。因此,在使用这个程序之前,请确保你清楚地了解其可能带来的影响,并谨慎操作。
相关推荐
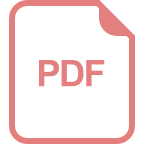
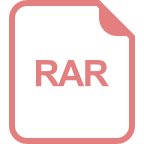
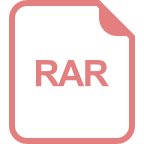













