c语言 通讯录管理系统
时间: 2023-09-02 19:08:49 浏览: 37
好的,通讯录管理系统可以分为添加联系人、删除联系人、修改联系人、查询联系人和显示所有联系人等功能。下面是一个简单的 C 语言实现:
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#define MAX_NAME_LEN 20
#define MAX_PHONE_LEN 15
#define MAX_CONTACTS_NUM 100
typedef struct {
char name[MAX_NAME_LEN];
char phone[MAX_PHONE_LEN];
} Contact;
int contacts_num = 0;
Contact contacts[MAX_CONTACTS_NUM];
void add_contact() {
if (contacts_num == MAX_CONTACTS_NUM) {
printf("The contacts list is full.\n");
return;
}
printf("Input name and phone number: ");
scanf("%s%s", contacts[contacts_num].name, contacts[contacts_num].phone);
contacts_num++;
printf("Contact added successfully.\n");
}
void delete_contact() {
char name[MAX_NAME_LEN];
printf("Input name to delete: ");
scanf("%s", name);
int i;
for (i = 0; i < contacts_num; i++) {
if (strcmp(contacts[i].name, name) == 0) {
int j;
for (j = i; j < contacts_num - 1; j++) {
strcpy(contacts[j].name, contacts[j + 1].name);
strcpy(contacts[j].phone, contacts[j + 1].phone);
}
contacts_num--;
printf("Contact deleted successfully.\n");
return;
}
}
printf("Contact not found.\n");
}
void modify_contact() {
char name[MAX_NAME_LEN];
printf("Input name to modify: ");
scanf("%s", name);
int i;
for (i = 0; i < contacts_num; i++) {
if (strcmp(contacts[i].name, name) == 0) {
printf("Input new phone number: ");
scanf("%s", contacts[i].phone);
printf("Contact modified successfully.\n");
return;
}
}
printf("Contact not found.\n");
}
void query_contact() {
char name[MAX_NAME_LEN];
printf("Input name to query: ");
scanf("%s", name);
int i;
for (i = 0; i < contacts_num; i++) {
if (strcmp(contacts[i].name, name) == 0) {
printf("Name: %s\nPhone: %s\n", contacts[i].name, contacts[i].phone);
return;
}
}
printf("Contact not found.\n");
}
void display_contacts() {
int i;
for (i = 0; i < contacts_num; i++) {
printf("Name: %s\nPhone: %s\n", contacts[i].name, contacts[i].phone);
}
}
int main() {
while (1) {
printf("Select an operation:\n");
printf("1. Add contact\n");
printf("2. Delete contact\n");
printf("3. Modify contact\n");
printf("4. Query contact\n");
printf("5. Display all contacts\n");
printf("6. Exit\n");
int choice;
scanf("%d", &choice);
switch (choice) {
case 1:
add_contact();
break;
case 2:
delete_contact();
break;
case 3:
modify_contact();
break;
case 4:
query_contact();
break;
case 5:
display_contacts();
break;
case 6:
printf("Goodbye!\n");
return 0;
default:
printf("Invalid choice.\n");
break;
}
}
}
```
这里使用了结构体来表示联系人信息,同时使用了动态数组来存储联系人列表。注意在添加联系人时要检查列表是否已满,删除联系人和修改联系人时要先查询联系人是否存在。
阅读全文
相关推荐
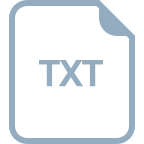
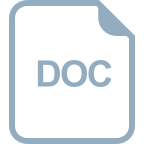
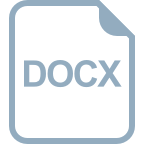
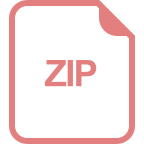
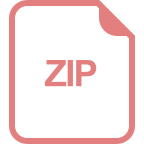
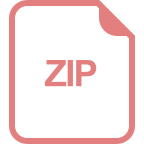