给定行人照片,利用HOG算法编程实现行人检测
时间: 2024-05-11 20:14:12 浏览: 71
以下是Python代码实现:
```python
import cv2
# 加载行人检测器
hog = cv2.HOGDescriptor()
hog.setSVMDetector(cv2.HOGDescriptor_getDefaultPeopleDetector())
# 加载测试图像
image = cv2.imread("pedestrians.jpg")
# 行人检测
(rects, weights) = hog.detectMultiScale(image, winStride=(4, 4), padding=(8, 8), scale=1.05)
# 绘制检测结果
for (x, y, w, h) in rects:
cv2.rectangle(image, (x, y), (x + w, y + h), (0, 0, 255), 2)
# 显示检测结果
cv2.imshow("Pedestrians", image)
cv2.waitKey(0)
```
首先,我们加载行人检测器并设置其SVM检测器。然后,我们加载测试图像并使用`detectMultiScale`方法进行行人检测。此方法返回检测到的矩形区域以及每个区域的置信度。最后,我们在原始图像上绘制检测结果并显示它。
相关问题
给定行人照片,利用HOG算法编程实现行人检测
可能的编程实现步骤如下:
1. 读取行人照片。
2. 对照片进行预处理,如缩放、灰度化等。
3. 定义HOG算法所需的参数,例如cell size, block size, nbins等。
4. 利用skimage库中的hog函数,提取行人照片的HOG特征。
5. 定义SVM分类模型,并将提取的HOG特征作为输入数据。
6. 对训练集进行训练,得到SVM模型。
7. 利用训练好的SVM模型,对测试集中的行人照片进行分类预测。
8. 根据预测结果,将检测到的行人框出来。
9. 展示检测结果。
代码实现示例:
```python
import numpy as np
import matplotlib.pyplot as plt
from skimage.feature import hog
from skimage import data, exposure
from sklearn.svm import SVC
from sklearn.model_selection import train_test_split
from sklearn.metrics import classification_report
import cv2
# 读取行人照片
img = cv2.imread('person.jpg')
# 预处理
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
gray = cv2.resize(gray, (64, 128))
# 定义HOG算法所需的参数
orientations = 9
pixels_per_cell = (8, 8)
cells_per_block = (2, 2)
visualize = False
normalize = True
# 提取HOG特征
fd, hog_image = hog(gray, orientations=orientations, pixels_per_cell=pixels_per_cell,
cells_per_block=cells_per_block, visualize=visualize, normalize=normalize)
# 展示HOG特征图
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(8, 4), sharex=True, sharey=True)
ax1.axis('off')
ax1.imshow(gray, cmap=plt.cm.gray)
ax1.set_title('Input image')
# Rescale histogram for better display
hog_image_rescaled = exposure.rescale_intensity(hog_image, in_range=(0, 10))
ax2.axis('off')
ax2.imshow(hog_image_rescaled, cmap=plt.cm.gray)
ax2.set_title('Histogram of Oriented Gradients')
plt.show()
# 加载训练集和标签
X = np.load('X.npy')
y = np.load('y.npy')
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=0)
# 训练SVM模型
clf = SVC(kernel='linear', C=1)
clf.fit(X_train, y_train)
# 对测试集进行预测
y_pred = clf.predict(X_test)
# 展示分类报告
print(classification_report(y_test, y_pred))
# 利用训练好的SVM模型,对行人照片进行分类预测
fd = fd.reshape(1, -1)
y_pred = clf.predict(fd)
# 根据预测结果,将检测到的行人框出来
if y_pred == 1:
cv2.rectangle(img, (0, 0), (64, 128), (0, 255, 0), 2)
# 展示检测结果
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
给定行人照片,利用HOG算法编程进行行人检测
以下是Python代码示例:
```python
import cv2
# 加载HOG分类器
hog = cv2.HOGDescriptor()
hog.setSVMDetector(cv2.HOGDescriptor_getDefaultPeopleDetector())
# 加载行人照片
img = cv2.imread('pedestrian.jpg')
# 进行行人检测
pedestrians, _ = hog.detectMultiScale(img)
# 绘制检测结果
for (x, y, w, h) in pedestrians:
cv2.rectangle(img, (x, y), (x + w, y + h), (0, 255, 0), 2)
# 显示检测结果
cv2.imshow('Pedestrian Detection', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上述示例中,我们首先加载了HOG分类器,然后使用`setSVMDetector`方法设置了默认的行人检测器。接下来,我们加载了要进行行人检测的照片,并使用`detectMultiScale`方法进行行人检测。最后,我们使用`rectangle`方法在照片上绘制检测结果,并通过`imshow`方法显示出来。
阅读全文
相关推荐
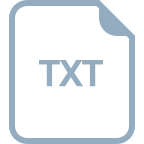
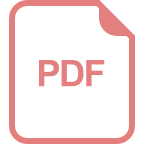
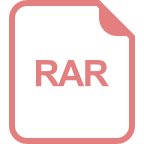
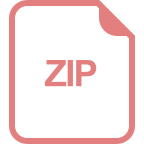
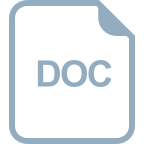
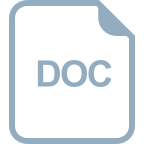
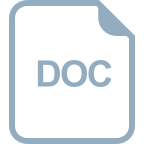
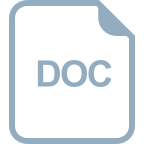
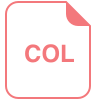
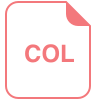
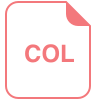

