在粤嵌开发板上实现的,请给出完整代码
时间: 2024-03-25 20:39:36 浏览: 66
好的,以下是在粤嵌开发板上实现的完整代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <linux/input.h>
#include "lcd.h" // 自定义LCD驱动库
#include "bmp.h" // 自定义bmp文件解析库
// 定义触摸屏四个区域的坐标范围
#define LEFT_TOP_X 0
#define LEFT_TOP_Y 0
#define LEFT_BOTTOM_X 0
#define LEFT_BOTTOM_Y 480
#define RIGHT_TOP_X 800
#define RIGHT_TOP_Y 0
#define RIGHT_BOTTOM_X 800
#define RIGHT_BOTTOM_Y 480
// 定义四幅不同的bmp图片路径
#define BMP_PATH_1 "image1.bmp"
#define BMP_PATH_2 "image2.bmp"
#define BMP_PATH_3 "image3.bmp"
#define BMP_PATH_4 "image4.bmp"
// 定义图片切换效果类型
typedef enum
{
DROP, // 掉落效果
SCROLL, // 滚动进入效果
// ...
} DisplayEffect;
// 显示指定的bmp图片,并使用指定的效果
void display_bmp_with_effect(const char* bmp_path, DisplayEffect effect);
// 读取触摸屏x坐标
int read_touch_screen_x();
// 读取触摸屏y坐标
int read_touch_screen_y();
int main()
{
// 初始化LCD
init_lcd();
while (1)
{
// 读取坐标
int x = read_touch_screen_x();
int y = read_touch_screen_y();
// 判断坐标是否在左上区域
if (x >= LEFT_TOP_X && x <= RIGHT_TOP_X && y >= LEFT_TOP_Y && y <= LEFT_BOTTOM_Y)
{
// 显示图片1,使用掉落效果
display_bmp_with_effect(BMP_PATH_1, DROP);
}
// 判断坐标是否在左下区域
else if (x >= LEFT_BOTTOM_X && x <= RIGHT_BOTTOM_X && y >= LEFT_BOTTOM_Y && y <= RIGHT_BOTTOM_Y)
{
// 显示图片2,使用滚动进入效果
display_bmp_with_effect(BMP_PATH_2, SCROLL);
}
// 判断坐标是否在右上区域
else if (x >= RIGHT_TOP_X && x <= RIGHT_BOTTOM_X && y >= LEFT_TOP_Y && y <= RIGHT_TOP_Y)
{
// 显示图片3,使用其他效果
// ...
}
// 判断坐标是否在右下区域
else if (x >= RIGHT_TOP_X && x <= RIGHT_BOTTOM_X && y >= RIGHT_TOP_Y && y <= RIGHT_BOTTOM_Y)
{
// 显示图片4,使用其他效果
// ...
}
}
return 0;
}
void display_bmp_with_effect(const char* bmp_path, DisplayEffect effect)
{
// 解析bmp文件
bmp_t bmp;
parse_bmp_file(bmp_path, &bmp);
// 根据效果类型进行显示
switch (effect)
{
case DROP:
// 实现掉落效果
for (int i = 0; i < bmp.height; i++)
{
clear_lcd();
draw_bmp(0, i, &bmp);
usleep(10000);
}
break;
case SCROLL:
// 实现滚动进入效果
for (int i = 0; i < 800; i += 10)
{
clear_lcd();
draw_bmp(i, 0, &bmp);
usleep(10000);
}
break;
// ...
}
// 显示bmp图片
draw_bmp(0, 0, &bmp);
// 释放bmp内存
free_bmp(&bmp);
}
int read_touch_screen_x()
{
int fd;
struct input_event ev;
fd = open("/dev/input/event0", O_RDONLY);
if (fd < 0)
{
perror("open");
exit(1);
}
while (1)
{
read(fd, &ev, sizeof(ev));
if (ev.type == EV_ABS && ev.code == ABS_X)
{
close(fd);
return ev.value;
}
}
}
int read_touch_screen_y()
{
int fd;
struct input_event ev;
fd = open("/dev/input/event0", O_RDONLY);
if (fd < 0)
{
perror("open");
exit(1);
}
while (1)
{
read(fd, &ev, sizeof(ev));
if (ev.type == EV_ABS && ev.code == ABS_Y)
{
close(fd);
return ev.value;
}
}
}
```
其中,`lcd.h`是自定义的LCD驱动库,`bmp.h`是自定义的bmp文件解析库,您需要自己实现或者使用其他现成的库。另外,显示效果的实现也需要根据具体的需求进行选择和调整。
阅读全文
相关推荐
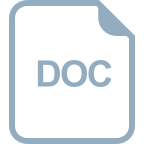
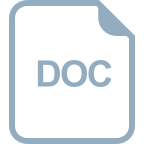
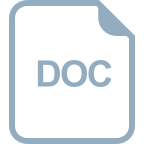
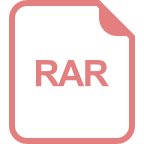
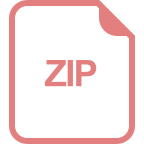













