python代码用pyqt实现鼠标点击画框
时间: 2024-06-01 21:02:14 浏览: 10
好的,这是一个关于Python代码实现鼠标点击画框的问题。我可以回答这个问题。你可以在PyQt中使用QPainter来实现这个功能。你需要创建一个QWidget并在其上调用mousePressEvent(),接着调用update()方法以便在屏幕上显示绘制的矩形。然后在paintEvent()中使用QPainter来绘制矩形。这是一个基本的框架,你可以尝试实现这个功能。
相关问题
pyqt5鼠标拖动图元移动 python代码
我可以回答这个问题。以下是一个简单的示例代码:
``` python
import sys
from PyQt5.QtWidgets import QApplication, QGraphicsScene, QGraphicsView, QGraphicsItem
from PyQt5.QtCore import Qt, QPointF
class CustomItem(QGraphicsItem):
def __init__(self, parent=None):
super(CustomItem, self).__init__(parent)
self.setFlag(QGraphicsItem.ItemIsMovable)
def boundingRect(self):
return Qt.QRectF(-50, -50, 100, 100)
def paint(self, painter, option, widget=None):
painter.drawRect(-50, -50, 100, 100)
if __name__ == '__main__':
app = QApplication(sys.argv)
view = QGraphicsView()
scene = QGraphicsScene()
item = CustomItem()
scene.addItem(item)
view.setScene(scene)
view.show()
sys.exit(app.exec_())
```
这个例子创建了一个自定义图元(CustomItem)并将其添加到QGraphicsScene中。该图元设置了标志ItemIsMovable以允许移动操作。在应用程序中运行代码后,你可以通过鼠标拖动CustomItem来移动它。
使用pyqt实现鼠标右键拖拽图片平移
以下是使用PyQt实现鼠标右键拖拽图片平移的简单示例代码:
```python
from PyQt5.QtCore import Qt
from PyQt5.QtGui import QPixmap
from PyQt5.QtWidgets import QApplication, QGraphicsScene, QGraphicsView
class ImageViewer(QGraphicsView):
def __init__(self, parent=None):
super(ImageViewer, self).__init__(parent)
# 创建场景
self.scene = QGraphicsScene(self)
self.setScene(self.scene)
# 加载图像
self.image = QPixmap('image.png')
# 添加图像到场景
self.image_item = self.scene.addPixmap(self.image)
# 开启拖拽模式
self.setDragMode(QGraphicsView.ScrollHandDrag)
# 重写鼠标按下事件
self.mousePressEvent = self.on_mouse_press_event
# 重写鼠标移动事件
self.mouseMoveEvent = self.on_mouse_move_event
def on_mouse_press_event(self, event):
# 如果是鼠标右键按下,则记录鼠标位置
if event.button() == Qt.RightButton:
self.last_mouse_pos = event.pos()
else:
super().mousePressEvent(event)
def on_mouse_move_event(self, event):
# 如果是鼠标右键移动,则计算偏移量并移动图像
if event.buttons() == Qt.RightButton:
offset = event.pos() - self.last_mouse_pos
self.image_item.moveBy(offset.x(), offset.y())
self.last_mouse_pos = event.pos()
else:
super().mouseMoveEvent(event)
if __name__ == '__main__':
app = QApplication([])
viewer = ImageViewer()
viewer.show()
app.exec_()
```
在这个示例中,我们重写了鼠标按下和鼠标移动事件。如果用户按下鼠标右键,则记录鼠标位置。在移动鼠标时,如果鼠标右键处于按下状态,则计算偏移量并移动图像。最后,我们将所有其他鼠标事件委托给父类处理。
相关推荐
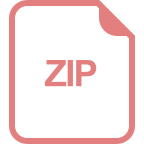
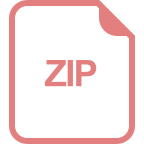
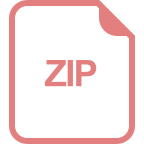
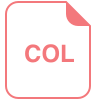
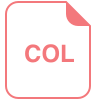
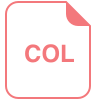
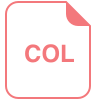
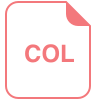







